Project: Ark
Ark is a desktop delivery management application that my team and I had built for our Software Engineering module in the National University of Singapore. We built this application for delivery companies to manage their deliveries. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 14kLoC. You may access the Ark repository here.
Ark was built on top of Addressbook - Level 4, an addressbook application. A number of features were added and refactoring was done to develop the original codebase into the parcel management application, Ark.
You may access the links below for a list of more significant contributions I have made to Ark. Functional code refers to the code that I have written to enhance the Ark application while Test code refers to the code that I have written to automate testing for the Ark application.
Code contributed: [Functional code][Test code]
The following are my contributions to the project explained in detail:
Autocomplete
Trying to type in long commands can be quite a hassle. That is why Ark comes with tab autocompletion which is able to help fill in commands as well as the prefixes for you without requiring you to fully type out the command with the needed fields. Ark autocompletion is smart and knows exactly what field you need, all you have to do is press Tab and let Ark handle the rest!
Autocomplete for commands:
To make use of the autocomplete feature for commands, simply type in the first few letters of the command you want
to use:
Figure 6.15.1 : Fill in the first few letters of the command you want to use. In this case, we want to use the Add
command, so we enter a
into the text field
now press the Tab key:
Figure 6.15.2 : After Tab is pressed Ark will fill in the command for you
Ark will then fill in the rest for you if there is a match.
If there is more than one possibility for the autocompletion, Ark will display the possible options to you as shown in the below.
Figure 6.15.3 : If there are multiple autocomplete options, the options will be displayed in the result window
In this case, you can press tab to cycle through the different options available.
Figure 6.15.4 : Pressing Tab again will cycle to the next matching command
Autocomplete for prefixes:
For commands that require prefixes, you can press Tab after filling in the command word. Ark will bring you to the
next missing prefix for you to fill in. After this, you can then press tab to cycle through the list of prefixes.
Figure 6.15.5 : After filling in the command into the text field, pressing Tab will bring you to the first missing prefix
Figure 6.15.6 : Pressing Tab will cycle to the next missing prefix
Alternatively, you can also enter the first letter of the prefix you want before you press Tab and Ark will fill
in the /
character for you.
Figure 6.15.7 : Fill in the command you want along with the first letter of the prefix you want
Figure 6.15.8 : Pressing Tab append the /
character for you
After you have chosen the prefix you want, simply enter in the details as needed.
Figure 6.15.9 : Fill in the needed details as per normal
After you are done entering the needed details of the prefix, press Tab to move on to the next prefix. Note that if your input ends with the first character of a prefix preceded by a space (e.g " n"), you will need to press Space before you press Tab to get the outcome you want.
Figure 6.15.10 : Pressing Tab will fill in the next missing prefix
Autocomplete for indexes:
For commands that require indexes, you can press Tab after filling in the command word. Ark will then
allow you to cycle through the indexes available by pressing Tab
Figure 6.15.11 : After filling in the command into the text field, pressing Tab will bring you to the first available index
Figure 6.15.12 : Pressing Tab will cycle to the next available index
Examples:
-
a
+ Tab (auto-completes withadd
in the command line input) -
e
+ Tab + Tab (cycles toexit
command) -
edit
+ Tab + Tab (auto-completes withedit 1
on the first tab and then cycles toedit 2
on the second tab) -
add
+ Tab + Tab (auto-completes withedit #/
on the first tab and then cycles toedit n/
on the second tab)
If you try to move on the the next prefix by pressing Space followed by Tab, Ark will still detect the
prefix as missing. Thus, the next prefix filled might be the same as the current prefix. e.g If the current text in the text field is add #/ you press Space followed by Tab, the result you will
get is add #/ #/ since the value of #/ has not been filled in yet.
|
End of Extract
Justification
Ark is an application that our users will be using on a regular basis. Therefore, a tab autocomplete feature will make it easier for our users to use the application and to improve their productivity. This increase in productivity is essential since Ark is meant to be used by people managing large volumes of deliveries each day.
Tab autocomplete mechanism
The tab autocomplete mechanism is facilitated by the autocompleter
package. The structure of the autocompleter
package can be seen in the class diagram below:
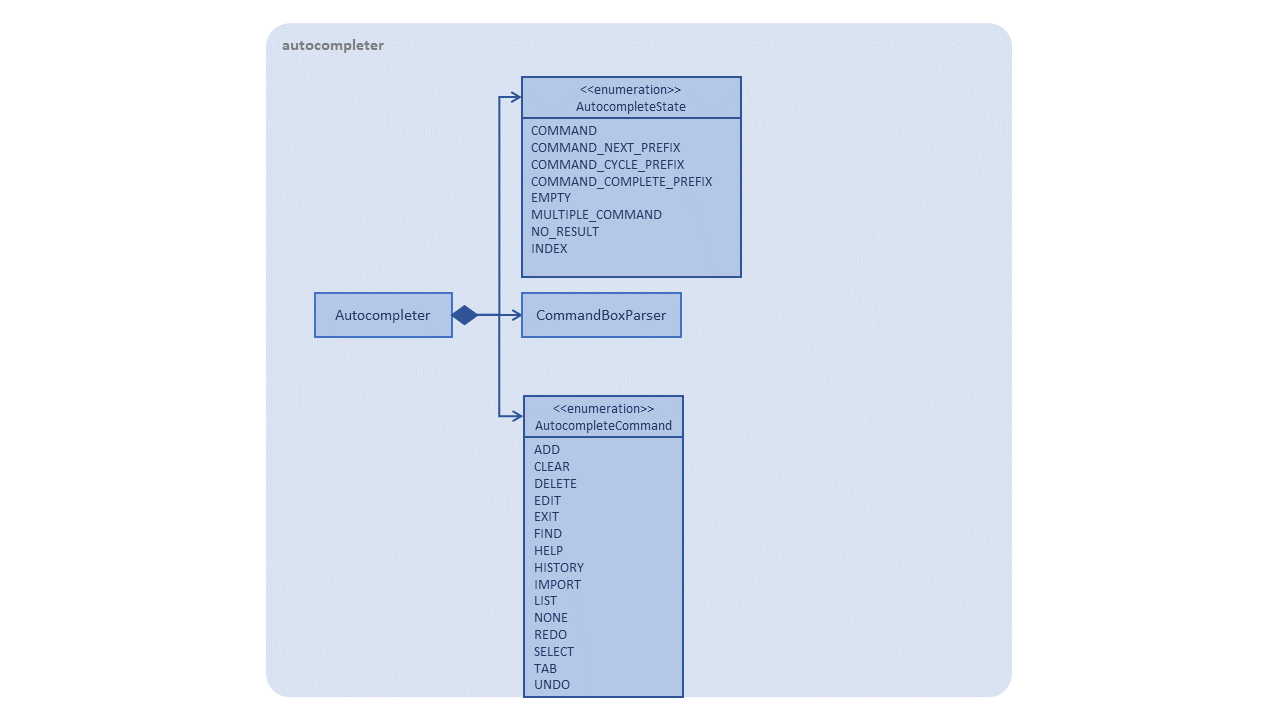
Figure 4.9.1 : Class diagram of the autocomplete package
The role of the CommandBoxParser
Class is to parse the text in the CommandBox
to extract commands and arguments as well
as to find missing prefixes.
The role of the AutocompleteCommand
Enum is to keep track of the current command that the Autocompleter
recognizes.
The rote of the AutocompleteState
Enum is to keep track of the current state of the Autocompleter
.
The Autocompleter
class is the main entry point into the package. An instance of the Autocompleter
class is
instantiated inside the CommandBox
class on start up. Inside the CommandBox
, an event listener is
attached to the TextField
which calls the updateAutocompleter
method whenever the text inside it is
changed. The updateAutocompleter
method then calls the updateAutocompleter
method in the Autocompleter
which
updates the state of the Autocompleter
according to the diagram below:
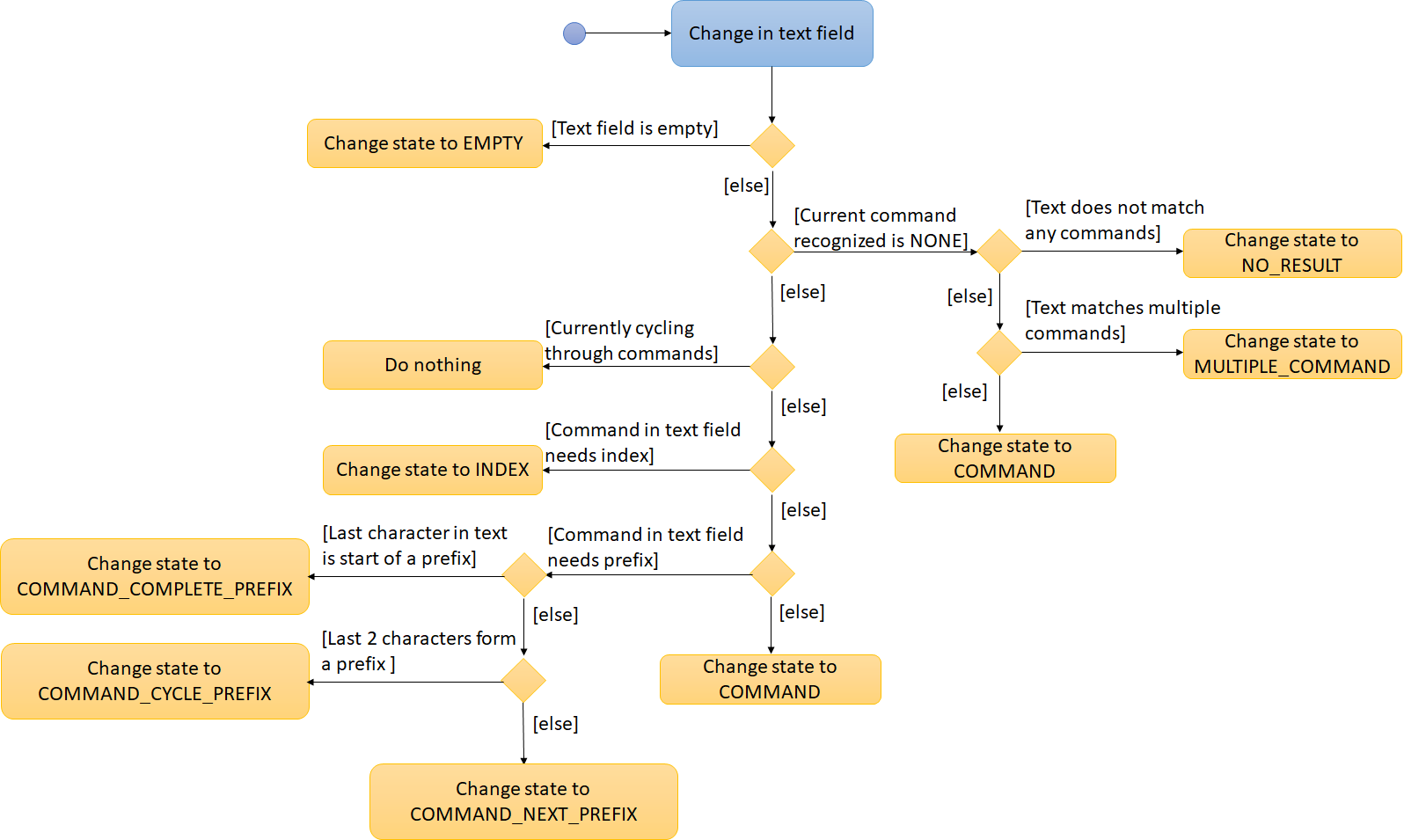
Figure 4.9.2 : Activity diagram of the updateAutocompleter
method
Besides updating the state, the updateState method also updates the possibleAutocompleteOptions
list. In the case
where there are multiple commands available, this will contain all the possible options. In the case where multiple
prefixes are available, this will contain the missing prefixes. These options are accessed using the resultIndex
which
is either incremented with wrap-around or reset depending on the state of the Autocompleter
when autocomplete
is called.
The countingIndex is used to keep track of the index field for commands that need it. Its maximum size is the
size of the current ActiveList in the model. It is either incremented with wrap-around or reset depending on
the state of the Autocompleter
when autocomplete
is called.
When tab is entered by the user, the autocomplete
method is called through the processAutocompelete
in the
Command box which then updates the text field according to the diagram below.
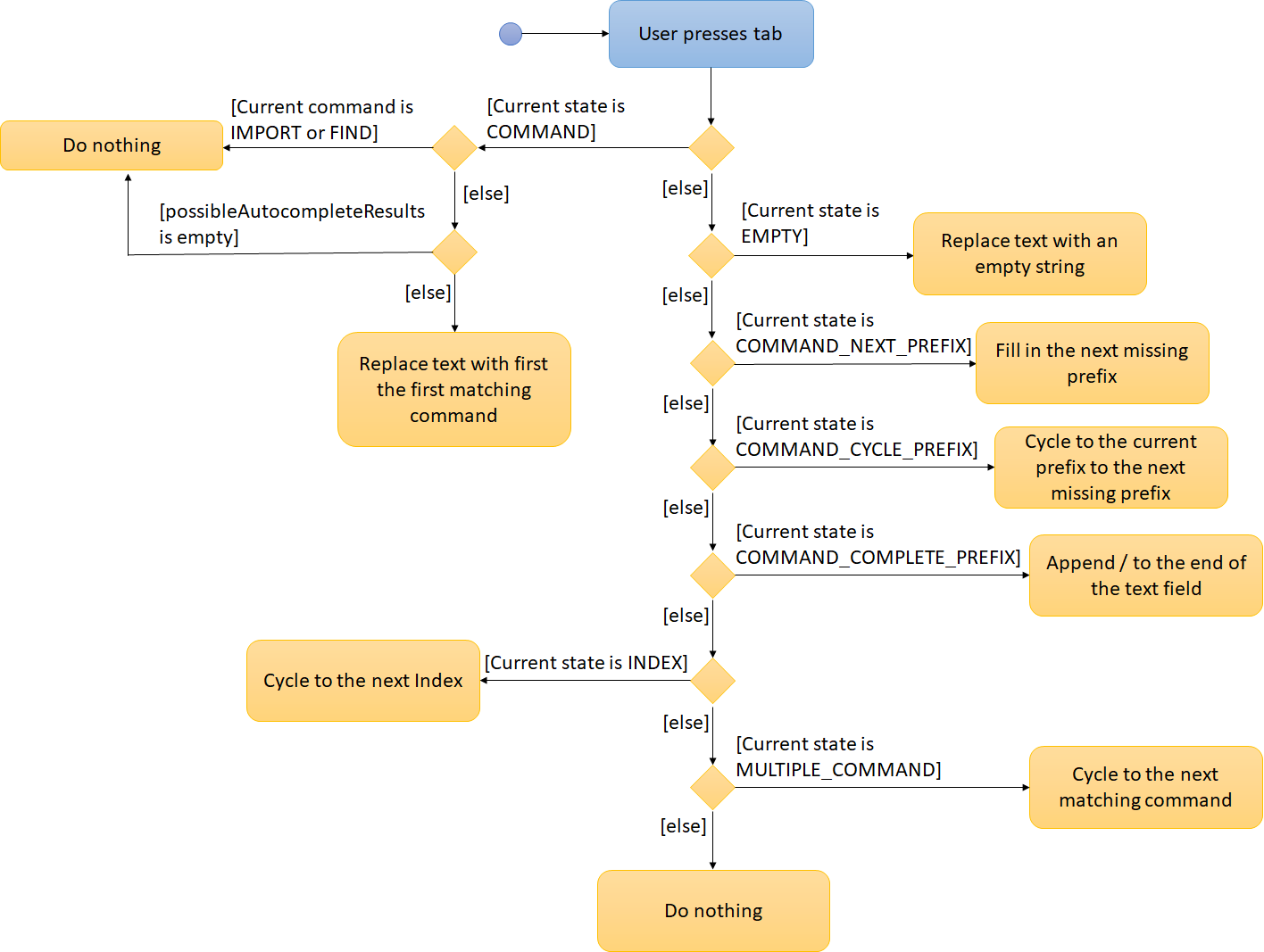
Figure 4.9.3 : Activity diagram of the autocomplete
method
Design Considerations
Aspect: autocomplete interface
-
Alternative 1 (current choice): A unix like tab auto-completion mechanism that allows users to cycle through options.
-
Pros: More intuitive and non-intrusive interface, which improves user experience.
-
Cons: Harder to implement, the whole autocompleter would be built from the ground up.
-
-
Alternative 2: A drop down box that gives users suggestions on the options they have.
-
Pros: Can be easily implemented by using a ComboBox in JavaFX.
-
Cons: Highly intrusive and isn’t as intuitive as a tab autocomplete. The ComboBox response time might also be too slow for people who type fast.
-
Aspect: Implementation of autocomplete
-
Alternative 1 (current choice): Create a new
Autocompleter
class to implementautocomplete
and its helper functions.-
Pros: Single Responsibility Principle (SRP) is maintained
-
Cons: More tedious to implement and test since the feature is implemented in both
Autocompleter
andCommandBox
. Also creates coupling between theAutocompleter
andCommandBox
.
-
-
Alternative 2: Implement
autocomplete
insideCommandBox
-
Pros: Easier to test since
CommandBoxTest
has already been set up and implemented. -
Cons:
CommandBox
class now has multiple responsibilities, which violates SRP.
-
End of Extract
Other contributions
-
Updated the add command to make Phone and email fields optional (Pull requests #114)
-
Wrote the Command Summary in the User guide as seen below:
Command Summary
Command |
Description |
Compulsory fields |
Optional fields |
Example usage |
Add |
Adds a new parcel to Ark |
|
|
|
Clear |
Deletes all parcels from Ark |
|
||
Delete |
Deletes a particular parcel from Ark |
|
|
|
Edit |
Edits the details of a particular parcel in Ark |
|
|
|
Find |
Searches Ark for a parcel containing a particular keyword |
|
|
|
List |
Lists all parcels currently in Ark |
|
||
Help |
Opens the help window |
|
||
Select |
Selects a particular parcel in Ark |
|
|
|
History |
Displays the history of previous commands executed in Ark |
|
||
Undo |
Undoes the latest command executed in Ark |
|
||
Redo |
Redoes the latest undone command in Ark |
|
||
Import |
Imports one or more parcels from an xml file |
|
|
Project: Modulus
Modulus is module mapping database to help NUS students in their search for possible modules in their Partner Universities that could possible be mapped back to modules back in NUS.