Project: Ark
Ark is a desktop delivery management application that my team and I had built for our Software Engineering module in the National University of Singapore. We built this application for delivery companies to manage their deliveries. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 14kLoC. You may access the Ark repository here.
Ark was built on top of Addressbook - Level 4, an addressbook application. A number of features were added and refactoring was done to develop the original codebase into the parcel management application, Ark.
You may access the links below for a list of more significant contributions I have made to Ark. Functional code refers to the code that I have written to enhance the Ark application while Test code refers to the code that I have written to automate testing for the Ark application.
Code contributed: [Functional code][Test code]
The following are my contributions to the project explained in detail:
Add Multiple Parcels into Ark from a Storage Fiel: import
You can import parcels into Ark using the import
feature. This feature works with valid save files of Ark. To use
this feature, you must first copy/move the save file that you want to import into the /data/import/
folder of
Ark’s home directory. Then you can type the following command into the CommandBox
, import
, followed by the name
of your file.
Ark will only import files that are stored in the .xml format.
|
Your file name is should only contain alphanumeric or underscore characters. e.g. ark_save , ark_save1 ;Files name that contain characters other than alphanumeric or underscore characters will be rejected. e.g. ark#1 ;Do not include the file type in your file name. The following inputs for the file name will be rejected. ark_save.xml ; |
Examples:
-
import ark
- This will import parcels stored indata/import/ark.xml
into the current instance of Ark -
import ark_save
- This will import parcels stored indata/import/ark_save.xml
into the current instance of Ark.
Format: import FILE_NAME
End of Extract
Justification
Parcel companies manage millions of deliveries on a daily basis. As such, they have to deal with large amounts of data and deliveries. Furthermore, adding parcels into Ark individually is time consuming. Hence, I built a feature that allows parcel companies to import parcels from storage files, and add parcels into Ark more efficiently.
Moreover, parcels are usually passed from one delivery company to another (or to another shipping company). Hence, I wanted to make it possible for delivery companies to be able to transfer their Ark storage files to each other as they transfer stewardship of parcels. This would save delivery companies time since they do not need to re-enter data that the previous delivery company had already entered.
I designed this feature to accept .xml
only files since Ark stores its files in .xml
format and I wanted the
import command to be compatible with its storage files so that it can import its storage files.
Import Mechanism
To use this command, you can type import
and the name of your file into the CommandBox
. e.g. import ark_save
The import
mechanism allows users to import parcels from valid storage files stored in a .xml
format. This mechanism
allows users to add multiple parcels stored in the imported storage file into the running instance of Ark. This
mechanism is facilitated by the readAddressBook()
method within AddressBookStorage()
to
load the parcels stored in the storage file and the ModelManager#addAllParcels
method to add the parcels in
the storage file into the running instance of Ark
.
Since the import
mechanism modifies the data stored in Ark
, it should be an extension of the UndoCommand
. Thus,
it inherits from the UndoableCommand
interface rather than inheriting direclty from the Command
interface.
The following sequence diagram describes the sequence of events that occur when you enter 'import ark_save' into the
CommandBox
:
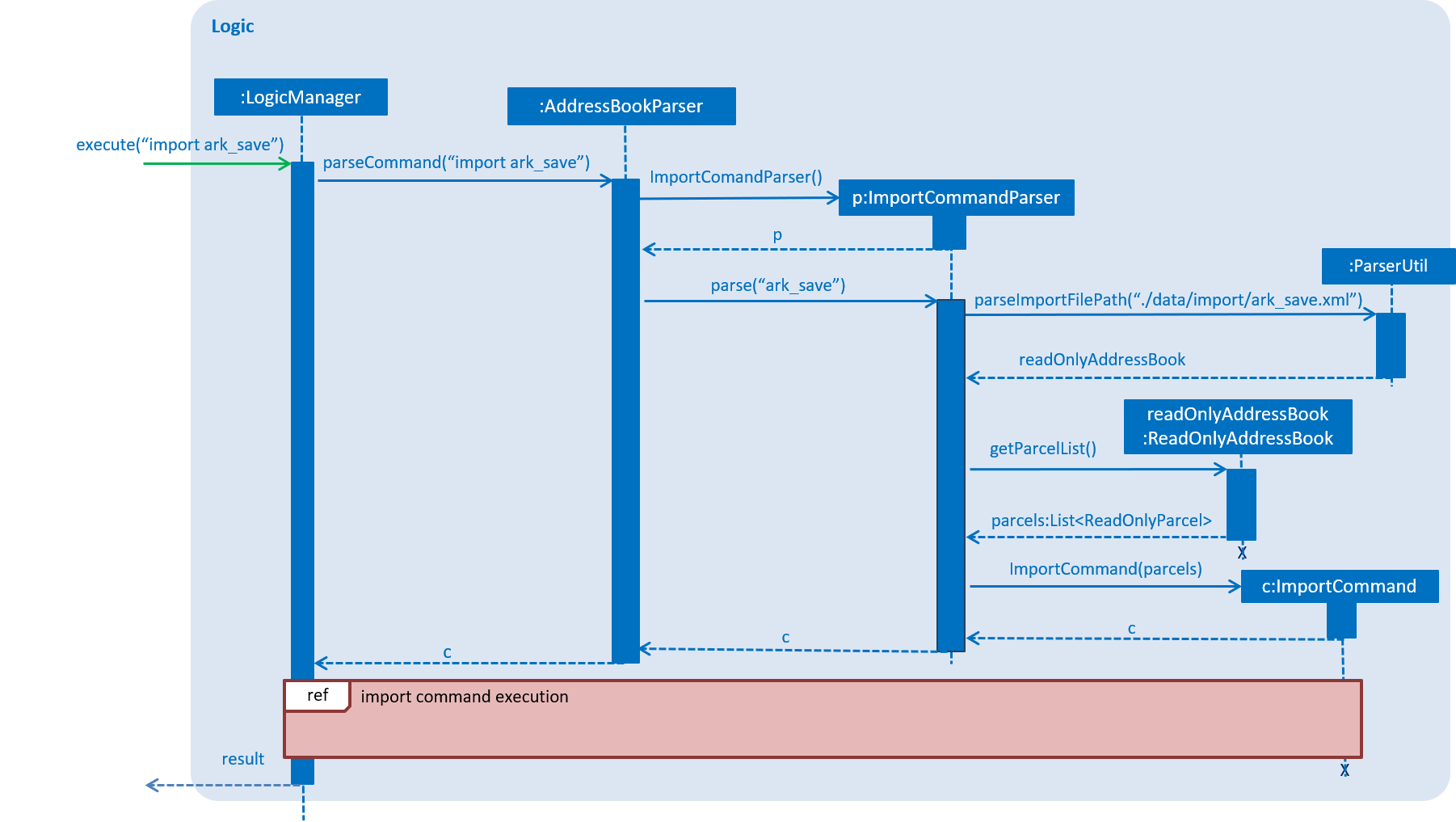
Figure 4.8.1 : Sequence diagram describing the operation of import
when it is executed
As seen in the sequence diagram above, the command is first parsed to create an ImportCommandParser
. This parser
takes the arguments of the import command ("ark_save")
as the name of the file to import and converts it to a
full file path string ("./data/import/ark_save.xml")
to locate the file to import. Thereafter, it loads the file
to import into Ark and reads the data. This returns a list of parcels that are used in create an ImportCommand
.
When the command is executed, the following sequence of events take place:
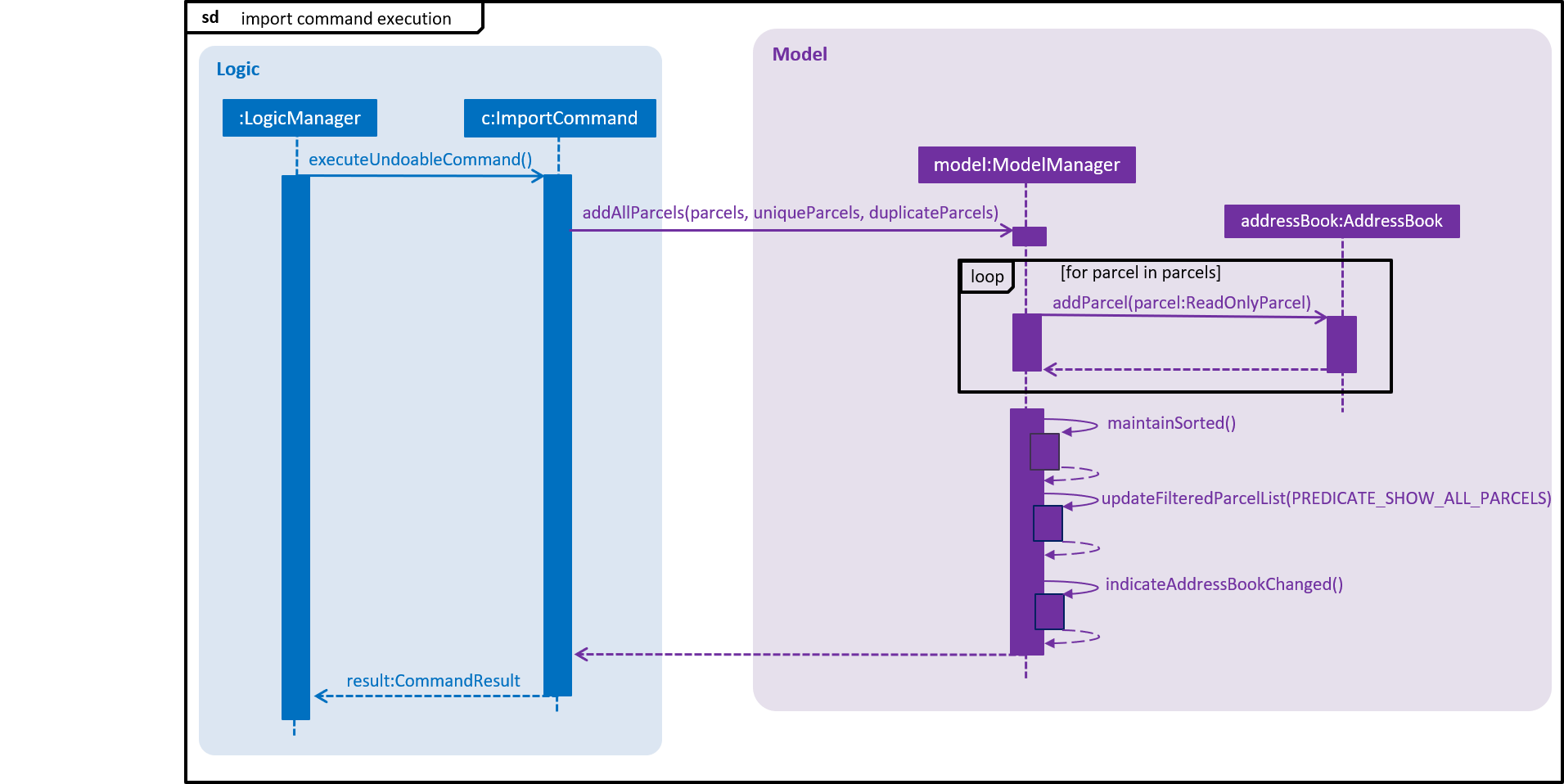
Figure 4.8.2 : Reference frame for the execution of the Import Command
As seen in the diagram above, when the command is executed, the executeUndoableMethod
methods calls
ModelManager#addAllParcels
method. In this method, all unique parcels are added into the running instance of Ark.
If the parcel is not unique such that it will create a duplicate parcel in the current instance of Ark,
the parcel is ignored and the process continues until the last parcel has been added.
The file to be imported has to be stored in the ./data/import folder. i.e. calling import ark_save will import the
file ./data/import/ark_save.xml .If the user enters a file name that contains characters other than alphanumeric characters or imports a file that is not in a .xml format, the command will throw an Exception.
|
The ImportCommand will only add non-duplicate parcels. Duplicate parcels are ignored.If all the parcels to be imported into Ark are duplicates, then no parcels are imported and an Exception is thrown. If the file to import is missing or empty, a CommandException will be thrown
|
Design Considerations
Aspect: Implementation of ImportCommand
-
Alternative 1 (current choice): using
readAddressBook()
to implement the logicImportCommand
**-
Pros: It becomes easier to implement method rather than writing out a separate logic to import files. It makes updates easier since enhancements to
readAddressBook()
will also enhance the import command such as more supported save file formats. -
Cons: This implementation increases the coupling of the
readAddressBook()
andImportCommand
such that changes inreadAddressBook()
is likely to cause a change inImportCommand
.
-
-
Alternative 2: Implement a parsing logic for
ImportCommand
.-
Pros: Reduced coupling of
readAddressBook()
andImportCommand
. This gives the developers more freedom on adding more file formats that can be imported. -
Cons: More tedious to implement and maintain
ImportCommand
since enhancements to thereadAddressBook()
feature has to be manually implemented inImportCommand
as well.
-
Aspect: Arguments to import files
-
Alternative 1 (current choice): Imports save files from only one location
-
Pros: User will only store his save files at one location, he will not store them at random locations and lose track of them. User only has to type the name of the file and does not need to type the full file path to locate the file. i.e. the user does not need to type
./data/import/Ark.xml
. -
Cons: The user has restrictions on where he can import files from.
-
-
Alternative 2: User can load the files from any directory
-
Pros: Allows user to import from his own archived folders anywhere in this computer.
-
Cons: More tedious for the user to type in the full file path to locate the .xml file that he wants to import.
-
Aspect: Allowed file names that can be imported
-
Alternative 1 (current choice): File Names can only contain alphanumeric and underscore characters and be in the
.xml
format.-
Pros: Users need to constraint their file naming to contain more semantic names rather than having non-alphanumeric or non-underscore characters in their naming of Ark save files.
-
Cons: The user has restrictions on the file naming conventions he can use to name the save files that he wants to import.
-
-
Alternative 2: No file name check
-
Pros: Allows user to name his files following any conventions and be successfully imported into Ark.
-
Cons: Makes Ark vulnerable to simple directory traversals where user can access files outside the
data/import/
directory.
-
End of Extract
Backing up the data
Ark data are backed up in the hard disk automatically at the start of every session of the program.
There is no need to back up the data manually.
The backup file is appended with -backup.xml
and is stored in the same folder as the main storage file.
To load the backup file into Ark, you can perform the following actions:
-
Firstly, open the
/data/
folder in the home directory of Ark. -
Then, copy the backup file, and paste the copied file into your
/data/import
directory. i.e.ark-backup.xml
-
Open the Ark Application. If Ark fails to start as a result of corrupted data in your Ark save file, simply delete the corrupted save file and restart the Ark application.
-
Finally, import the backup save file into Ark by using the
import
command by typingimport (back-up file name)
. i.e.import ark-backup.xml
Alternatively, you can delete your original save file and rename your backup file to the name of your original save file. However, this approach will result in data being loss if your original save file was not corrupted and contains valuable data.
If Ark is unable to read your save file for reasons such as the save file being of an invalid format or if your save
file was missing (gasp), Ark would not create a backup file for you on start up. |
End of Extract
Justification
Delivery information is very important to delivery companies. Since it is impossible to prevent corruption of storage files, I developed an alternative measure, a feature that automatically backs up a reasonable recent version of the corrupted save file.
Backup Mechanism
The back up mechanism is facilitated by a backup(addressBook:AddressBookStorage)
method within the StorageManager
class. It supports the backup of data in Ark.
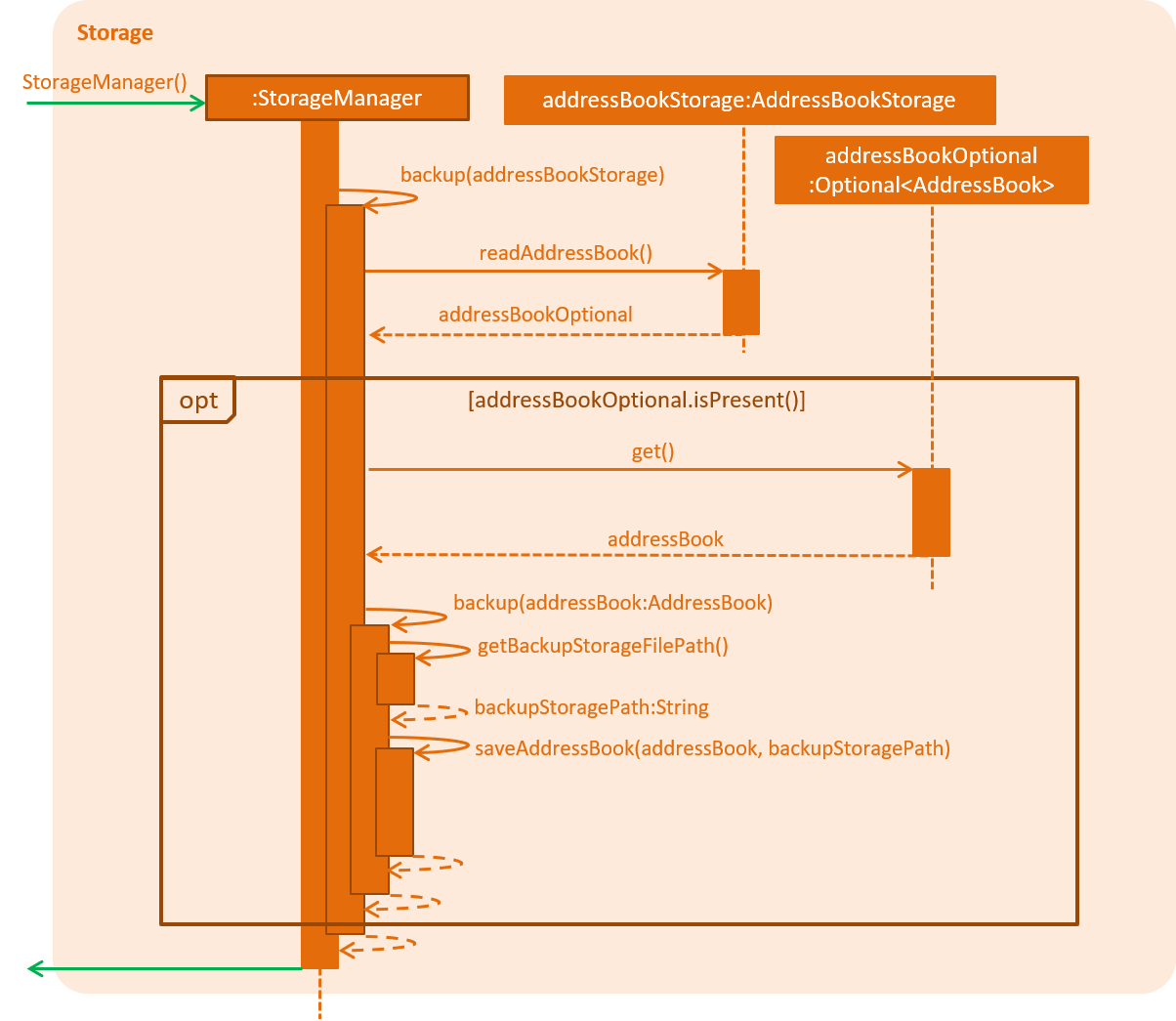
Diagram 4.7.1 : Sequence diagram describing the operation of the storageManager
when it is initialized
As seen in the sequence diagram above, the backup(addressBook:AddressBookStorage)
method is called when
storageManager
is initialised in MainApp#init()
. The MainApp#init()
is called when Ark launches.
From the diagram above, the backup()
method is called when storageManager
is initialized. This backup method first
checks if there is a valid save file loaded into Ark. If there is a save file loaded into Ark,
the backup(addressBook:AddressBook)
method will be called. Otherwise, Ark does not backup the missing save file.
To save the backup data, Ark first retrieves the backup file path by calling the getBackupStorageFilePath()
method.
Then, it will call the saveAddressBook(addressBook, backupStoragePath)
method to save a backup of Ark at the retrived
backup file path.
Design Considerations
Aspect: Implementation of StorageManager#backup()
-
Alternative 1 (current choice): use the
saveAddressBook()
method to implement logic.-
Pros: It becomes easier to implement method rather than writing out a separate logic for
backup()
. It makes updates easier since enhancements to saving Ark insaveAddressBook()
will also enhancebackup()
. -
Cons: This implementation increases the coupling of
backup()
andsaveAddressBook()
where changes insaveAddressBook()
are likely to cause changes inbackup()
.
-
-
Alternative 2: Separate the implementation of
backup()
fromsaveAddressBook()
-
Pros: Reduced coupling of
saveAddressBook()
andbackup()
and allows the backup file to be saved at a different location from the main save file. This prevents the backup file from being corrupted if the folder of the main save file becomes corrupted. -
Cons: More tedious to implement and maintain
backup()
since enhancements to the saving feature has to be implemented in bothsaveAddressBook()
andbackup()
-
Aspect: Trigger to execute the backup mechanism
-
Alternative 1 (current choice): Automatically backup data on launch.
-
Pros: This implementation ensures that the if the user corrupts the data of Ark during a session. The user will be able to revert to the start of the session, which is likely to be a functional copy of his original save file.
-
Cons: This does not give the most recent copy of the data of the Ark if many changes were made in a single session.
1* Alternative 2: Backup data every few minutes -
Pros: Provides a very recent copy of the data on Ark.
-
Cons: More tedious and difficult to implement. User may also be running another process at that point of time. This could cause a bottleneck if there is a lot of data to be saved, and multiple backup calls will be queued one after the other if the previous backup process is called even before the current one has finished running.
-
-
Alternative 3: Backup data after a fixed number of
UndoableCommand
.-
Pros: Provides a very recent copy of the data on Ark.
-
Cons: More tedious and difficult to implement. Difficult to determine the optimal amount of data to restore. If the corruption of the data is caused by a series of commands, it becomes difficult to provide reasonable assurance that the backup file provides a functional copy of the data of Ark.
-
Aspect: Case: Backup if main storage file is not present
-
Alternative 1 (current choice): Ark does not backup if it cannot read the main storage file
-
Pros: Backup data will not be overwritten in the event that Ark is not able to read the designated save file for reasons such as the save file being corrupted/missing.
-
Cons: Additional overhead to check if Ark is able to read the save file.
-
-
Alternative 2:Back up even if the main storage file does not exist or cannot be read by Ark.
-
Pros: Less overhead needed to check if Ark is able to read the save file.
-
Cons: Backup data could be overwritten in the event the designated save file is corrupted/missing.
-
End of Extract
Status Field: Delivery Status of Parcel
Status
is used to indicate the current delivery status of a parcel. A parcel can have 4 possible delivery status and
listed below is a description of these Status
values.
Status | Description |
---|---|
PENDING |
This means that the parcel has not been delivered and has not passed the date it is supposed to be delivered by. |
DELIVERING |
This means that the parcel is currently working being delivered to its destination address. |
COMPLETED |
This indicates that the parcel has been successfully delivered to its destination. |
OVERDUE |
This state indicates that the parcel has not been delivered and has passed its due date. |
To input a Status
, you can type case-insensitive formats of the above Status. e.g. pending
or Pending
are valid
inputs to add a PENDING
Status
.
Values other than the above values will be rejected. e.g. PROCESSING , PROCESSED ;
|
End of Extract
Justification
Parcels can be in several stages of a delivery process. They can be pending delivery, or being delivered at a particular instance. Hence, it is imperative that delivery companies are able to assign a status to their parcels to have a clear idea of the stage of delivery that their parcels are at. Moreover, it allows delivery customers to be accountable to their clients when their clients inquire about the delivery status of their parcels.
In addition, this feature has an automatic Status
update base on the delivery date of the parcel. If the delivery date has
been passed, the parcel Status
updates to OVERDUE
. Otherwise, it will update to PENDING
. This update only works
for parcels that have PENDING
or OVERDUE
Status
. The automatic update feature allows our users to have a more
intuitive description of the urgency of a parcel delivery. Rather than observing the date to determine if the parcel is
overdue, Ark’s Status
feature, with its automatic updates helps users visualize this information more intuitively.
Status field
Status
represents the current stage of delivery that a parcel is at. As seen in the class diagram below,
Status
implements an Enumeration
interface and it has the four possible values:
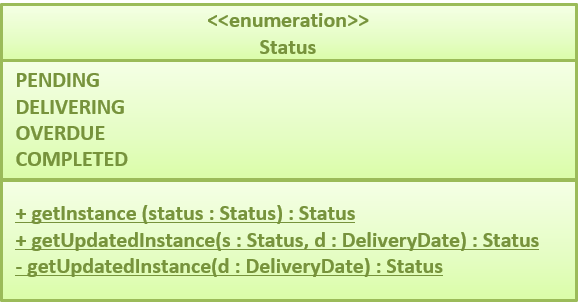
Figure 4.12.1: Status Class Diagram
The following are the descriptions for the four possible values of Status
:
Status | Description |
---|---|
PENDING |
This means that the parcel has not been delivered and has not passed the date it is supposed to be delivered by. |
DELIVERING |
This means that the parcel is currently working being delivered to its destination address. |
COMPLETED |
This indicates that the parcel has been successfully delivered to its destination. |
OVERDUE |
This state indicates that the parcel has not been delivered and has passed its due date. |
As seen in the class diagram, the getInstance()
method retrieves the static instance of Status
based on the status
String input. For example, getInstance("pending")
will return the PENDING Status
. Additionally, Status is updated
automatically when a parcel is edited or added. The Status
of parcels in Ark will also be updated when Ark first launches.
This is done through the getUpdatedInstance(s : Status, d : DeliveryDate)
method and the overloaded method
getUpdated(d : DeliveryDate)
method.
The automatic Status
update updates based on the comparison of today’s date to the DeliveryDate
object given as the
parameter of getUpdatedStatus()
. If today’s date is after the date indicated in the DeliveryDate
object, the method
will return an OVERDUE Status
. Otherwise, it will return a PENDING Status
. The Status
update only works for
Status
values of PENDING
and OVERDUE
. COMPLETED
and DELIVERING Status
are not updated.
Design Considerations
Implementation of Status
-
Alternative 1 (current choice): Status implements an Enumeration interface.
-
Pros:
Status
has a fixed number of values. The user should also not be allowed to create newStatus
objects. This also standardizes the naming conventions used to describe the same delivery status in Ark. -
Cons: Less options for the user to alter the
Status
values
-
-
Alternative 2: Allow the user to define any
Status
they wish.-
Pros: Users have more versatility on naming conventions
-
Cons: It becomes more difficult to import data files since different users may use different terminologies to describe the same
Status
of the parcel.
-
Updating of Status
-
Alternative 1 (current choice): Separate
Status
update from retrieving an instance of Status-
Pros: By separating the update and retrieval, we are using the Separation of Concerns Principle,
getInstance()
checks if the String input is a validStatus
and returns it. On the other hand,getUpdatedInstance()
receives a validStatus
and returns an updatedStatus
with respect to theDeliveryDate
provided as a parameter. Easier to test these methods and integrate the automaticStatus
update into the codebase. -
Cons: More overhead.
-
-
Alternative 2: Update
Status
ingetInstance()
.-
Pros: Less overhead and more intuitive.
-
Cons: More tedious to code because a
DeliveryDate
object has to be instantiated before user is able to retrieve an updatedStatus
.
-
End of Extract
Tracking Number Field: Tracking Number of Parcel
The Tracking Number field represents the tracking number of the parcels.
This field allows you to add tracking numbers to your parcels. Tracking numbers allow you to keep track of the parcels that are in your possession. This field is important because a single person can have many parcels belonging to him. Hence, you can use our tracking number system to differentiate between the different parcels that are allocated to a single recipient. You can also use tracking numbers to identify specific parcels.
Ark only supports tracking numbers for registered articles managed by SingPost at this instance. These
numbers include two R`s followed by nine digits and ending with `SG . e.g. RR123456789SG You can read more about SingPost Registered Article tracking number here. Tracking numbers that do not conform to the above format will be rejected e.g. rr123545679sg ; RR#12345678SG ;
|
You can add multiple parcels with the same tracking number. This allows you to reuse tracking numbers in the event when
the number of parcels in your inventory exceeds the number of tracking numbers. There has been instances of this
occurring during festive seasons such as Christmas. The team is working on adding support for more types of tracking numbers such as those of UPS and FedEx in the future. |
End of Extract
Justification
Delivery companies use tracking numbers to track their parcels in their possession. Tracking numbers are necessary since a single person can have many different items that are meant to delivered to him on a single day. Tracking numbers help our users and their clients differentiate between these parcels. This helps our users become more accountable to clients if a certain parcel has not been delivered as well.
Tracking Number Field
Parcels have tracking numbers for delivery vendors to keep track of the parcels that they send out on a daily basis. This feature is important because a single person can have many parcels belonging to him. Tracking numbers are used to differentiate between the different parcels that are going to be delivered to the same person. Tracking numbers also serve as a better way of narrowing down and pinpointing parcels of interest since these numbers are likely to be more unique than names in a localized region.
Presently, the Tracking Number Field only has support for Registered Article tracking numbers belonging to SingPost.
You can read more about their Registered Article tracking number
here.
|
Design Considerations
Tracking Numbers implementation.
-
Alternative 1 (current choice): Ark allows duplicate
TrackingNumber
entries.-
Pros: In the event that the number of parcels in the users' inventory exceeds the number of possible
TrackingNumber
entries, the user will still be able to add the parcel into Ark. There has been instances where tracking numbers were reused. Moreover, different delivery companies have different policies on how the tracking numbers` are used. Therefore, it is difficult to implement a general policy forTrackingNumber
entries. -
Cons: Less intuitive to users.
-
-
Alternative 2: Ark only allows non-duplicate
TrackingNumber
entries.-
Pros: More intuitive for users.
-
Cons: Impossible to add parcel with duplicate entry even if the parcel does have that specific
Tracking Number
. This issue can occur during festive periods such as Christmas when the number of parcels can exceed the number ofTrackingNumber
entries.
-
End of Extract
Enhancement Added: Postal Code Feature
Postal Code: Postal Code of Delivery Address of the Parcel
Ark can store the postal address of locations in Singapore. It only accepts values of s
or S
followed by 6 digits.
The postal code of a parcel is used to query Google Maps when the select
command is executed or when a parcel in the
Parcel List Panel is clicked. Invalid values would include the following: 213661
; s#11122
;
Postal codes must be appended to the end of the address text. e.g. Suppose you are adding a new parcel into Ark and
entered add …(Some info)… a/NUS School of Computing, COM1, 13 Computing Drive, S117417 …(More info required
in the add command)…
. In this case, S11417
is the postal code of the above address and is appended to its
respective address, separated by a space.
If you did not append the postal code of the address at the end of your address, Ark will not recognize your address input as a valid input and would give you an invalid input message. |
Presently, the PostalCode field still does a very relaxed validation and does not completely ensure that the postal
code exists even though it might meet the criteria above. The team is working on producing a database of postal codes
in Singapore. In the meantime, we encourage users to take additional precautions when entering the postal codes
into Ark and ensure that the postal code inputs are valid.
|
End of Extract
Justification
Addresses in Singapore have a postal code representation. We use this postal code feature to query Google Maps on the destination address of a parcel and help our users visualize this information. This feature is necessary because addresses in Singapore can have similar names (Type OCBC into Google Maps, and you will observe that OCBC has many branches) around Singapore. Hence, postal codes will help Google Maps pinpoint the exact address that we should deliver a parcel too. Furthermore, postal codes are short and succinct and do not require an extensive amount of effort for the user to input this data.
Postal Code Field
The postal code field is represented using the PostalCode
class. The PostalCode
field is implemented as part of the
Address
class. The PostalCode
class stores the postal address the address text. It only accepts values of
s
or S
followed by 6 digits. The PostalCode
will generate a String
to query Google Maps when the select
command is executed or a when a parcel is selected.
Presently, the PostalCode field still does a very relaxed validation and does not completely ensure that the postal
code exists even though it might meet the criteria above. The team is working on producing a database of postal codes
in Singapore by querying the Google Maps Distance Matrix API. In the meantime, it is assumed that users will enter
the correct postal code.
|
Design Considerations
Implementation of Postal Code
-
Alternative 1 (current choice):
Postal Code
is designed as a part of theAddress
class.-
Pros: This composition relationship is more intuitive. If an
Address
is deleted, its correspondingPostalCode
is also deleted. -
Cons: -
-
-
Alternative 2: Separate the
PostalCode
class from theAddress
class-
Pros: -
-
Cons: When an
Address
is deleted, its correspondingPostalCode
has to be searched and deleted as well. This results in more overhead.
-
Valid inputs to the PostalCode
class
-
Alternative 1 (current choice): `Postal code accepts postal district codes above 80. (first two digits of postal code)
-
Pros: This ensures that when new postal code districts are added into Singapore, the user will be able to add the postal codes from the new postal code district without receiving an error.
-
Cons: The user might enter postal codes that belong to a non-existent district e.g. S810000
-
-
Alternative 2: Reject postal codes with postal district codes above 80.
-
Pros: The user will have a stricter validation of his postal code.
-
Cons: If a new postal district is added, e.g. 81, and there is parcel that has a postal code belonging to the district, Ark will reject that parcel from being entered,
-
End of Extract
Tags Field: Delivery Tags of Parcel
Tags
are used to indicate how the parcel should be handled. Tags can contain one or more of the following Tag
:
Tag | Description |
---|---|
FROZEN |
This means the parcel should be refrigerated as its contents are temperature sensitive. |
FLAMMABLE |
This means that the parcels' contents are highly flammable and should be kept away from heat. |
HEAVY |
This indicates that the parcel is heavy and may require additional manpower to deliver. |
FRAGILE |
This state indicates that the parcels' contents can be broken easily and requires additional care when handling. |
To input a Tag
, you can type insensitive formats of any of the above Tags
. e.g. frozen
or Frozen
are valid inputs
to add a FROZEN
Tag
.
Values other than the above values will be rejected. e.g. friends , colleagues .
If your use of Ark requires more tags to be made available, please contact our team and we will see to your request.
|
End of Extract
Justification
More often than not, different parcels require different types of handling. The Tag feature is used to help users tag parcels with tags to describe how the parcel needs to be handled. This information helps to ensure that proper handling precautions are taken while handling a parcel and ensures that it reaches its intended recipient safely.
Tags Field
Tags
are used to indicate how the parcel should be handled. The Tags
field can contain one or more of the following Tag
:
Tag | Description |
---|---|
FROZEN |
This means the parcel should be refrigerated as its contents are temperature sensitive. |
FLAMMABLE |
This means that the parcels' contents are highly flammable and should be kept away from heat. |
HEAVY |
This indicates that the parcel is heavy and may require additional manpower to deliver. |
FRAGILE |
This state indicates that the parcels' contents can be broken easily and requires additional care when handling. |
Design Considerations
Implementation of Tag
-
Alternative 1 (current choice): Tag implements the Enumeration interface.
-
Pros:
Tag`s has fixed values. The user should also not be allowed to create new `Tag
objects. -
Cons: Less options for the user to alter the
Tag
values
-
-
Alternative 2: Allow the user to define any `Tag`s they wish.
-
Pros: Users have more versatility on naming conventions
-
Cons: It becomes difficult for delivery personnel to keep track of the tags since different personnel might use different tag names to refer to the same tag.
-
End of Extract
Enhancement Added: Tooltip Feature
Parcel Tooltip
Ark comes with a tooltip feature that allows you to view parcel details that are too long. Suppose you have entered
a parcel with really long details into Ark. As seen below, John’s parcel has a delivery address that is too long,
and a part of it has been replaced by ellipsis i.e. …
as seen in figure 4.21.1 below.
Figure 4.2.1.1 : Parcel with a long address
To view the full delivery address, we simply mouseover his address and the full details of his address will appear as a tooltip. This can be seen in figure 4.22.2 below.

Figure 4.2.1.2 : Tooltip to show more of John’s address
End of Extract
Justification
There are occasions where inputs to Ark are really long. To ensure that our users will be able to see the full input in Ark, a tooltip is added to help our users visualize this elongated data as observed in the diagram above.
GUI tab mechanism
To help you organise between the parcels you have delivered and the parcels you have delivered, Ark provides you two lists, one containing the parcels you have yet to deliver, and the other, the parcels that you have already delivered.
When you launch the application, Ark will show the list of undelivered parcels in your inventory. To view
the list of delivered parcels, you can click on the Completed Parcel
tab located on the Tab Panel of Ark.
Figure 4.3.1 : Clicking on Completed Parcel
tab
As seen above in figure 4.3.1, the list has changed to the list of parcels that have been delivered. To revert back to the list of
parcels that have not been delivered, simply click on the All Parcels
tab.
Parcels that have a COMPLETED status will be added to the list of delivered parcels directly while Parcels
that do not have a COMPLETED status will be added to the list of undelivered parcels.
|
End of Extract
Justification
Users can use this feature to segment the work (parcels) that have completed and the parcels they have to yet to deliver. This helps users to differentiate between the tasks they have completed and tasks that they have to do. By organising this data into separate lists, we can help users to visualize information about their parcels more intuitively without having a clunky interface.
Documentation Contributions
Here are some of the more significant segments of documentation that I’ve written for the Ark project.
Parcel Fields
Name Field: Name of Parcel Recipient
The Name field represents the name of the parcel’s recipient. It can contain the name of the organisation or the person
that you are delivering to the parcel to.
The Name field will only allow alphanumeric characters separated by whitespace. e.g. John Doe ;
8 Noodles at Shangri-La’s Rasa Sentosa Resort & Spa Non-alphanumeric entries will be rejected by Ark. e.g. John Doe!
|
Tracking Number Field: Tracking Number of Parcel
The Tracking Number field represents the tracking number of the parcels.
This field allows you to add tracking numbers to your parcels. Tracking numbers allow you to keep track of the parcels that are in your possession. This field is important because a single person can have many parcels belonging to him. Hence, you can use our tracking number system to differentiate between the different parcels that are allocated to a single recipient. You can also use tracking numbers to identify specific parcels.
Ark only supports tracking numbers for registered articles managed by SingPost at this instance. These
numbers include two R`s followed by nine digits and ending with `SG . e.g. RR123456789SG You can read more about SingPost Registered Article tracking number here. Tracking numbers that do not conform to the above format will be rejected e.g. rr123545679sg ; RR#12345678SG ;
|
You can add multiple parcels with the same tracking number. This allows you to reuse tracking numbers in the event when
the number of parcels in your inventory exceeds the number of tracking numbers. There has been instances of this
occurring during festive seasons such as Christmas. The team is working on adding support for more types of tracking numbers such as those of UPS and FedEx in the future. |
Phone Field: Phone of Parcel Recipient
The Phone field represents the phone number of the parcel’s recipient. It can contain the phone number of the
organisation or the person that you are delivering to the parcel to.
You can only assign a single phone number to a each parcel. You may omit this field in your entry, preferably only
when it is not provided.
The Phone field will only allow you to add phone numbers with 3 or more digits. e.g. 1234 5678 ; 1122 2344 5678 ;This is to allow you to add overseas numbers per the request of the parcel’s recipient. Phone numbers shorter than 3 digits will be rejected. e.g. 4 , 12 ; |
Address Field: Destination Address of Parcel Delivery
The Address field represents the destination address of your parcel. This field contains the address that you should deliver your parcel to.
The Address field will only allow you to add an address with at least a single character as the text representation of
the address, and appended with a valid postal code, separated from the text representation of the address with space(s).
e.g. 123, example street #05-26 S012345 ;Invalid address entries include the following: e.g. 123, example street #05-26
You can learn more about valid postal code entries below. |
Postal Code: Postal Code of Delivery Address of the Parcel
Ark can store the postal address of locations in Singapore. It only accepts values of s
or S
followed by 6 digits.
The postal code of a parcel is used to query Google Maps when the select
command is executed or when a parcel in the
Parcel List Panel is clicked. Invalid values would include the following: 213661
; s#11122
;
Postal codes must be appended to the end of the address text. e.g. Suppose you are adding a new parcel into Ark and
entered add …(Some info)… a/NUS School of Computing, COM1, 13 Computing Drive, S117417 …(More info required
in the add command)…
. In this case, S11417
is the postal code of the above address and is appended to its
respective address, separated by a space.
If you did not append the postal code of the address at the end of your address, Ark will not recognize your address input as a valid input and would give you an invalid input message. |
Presently, the PostalCode field still does a very relaxed validation and does not completely ensure that the postal
code exists even though it might meet the criteria above. The team is working on producing a database of postal codes
in Singapore. In the meantime, we encourage users to take additional precautions when entering the postal codes
into Ark and ensure that the postal code inputs are valid.
|
Email Field: Email of Parcel Recipient
The Email field represents the email contact of the parcel’s recipient. It can contain the email of the organisation or the person that you are delivering to the parcel to.
The Email field will only allow alphanumeric or periods characters separated by an @ character. e.g. John@example.com
Invalid Email entries include the following: e.g. John_Doe@example.com ; JaneDoe.example.com ;
|
Due Date Field: Due Date for Parcel Delivery
Delivery Date
is used to indicate the delivery date that the parcel must be delivered by.
The dates are only accepted if they are in the valid format DD-MM-YYYY or understandable by Ark.
Ark is able to recognise various forms of dates as shown in the table below but the dates in the Ark are formatted as DD-MM-YYYY. However, invalid inputs such as a phone number or symbols still will be rejected.
Current date as of writing is 12 November 2017.
User input | Date parsed by Ark |
---|---|
01-01-2017 |
01-01-2017 |
01/01/2017 |
01-01-2017 |
01.01.2017 |
01-01-2017 |
01-01-17 |
01-01-2017 |
First day of 2017 |
01-01-2017 |
The day before yesterday |
10-11-2017 |
Yesterday |
11-11-2017 |
Today |
12-11-2017 |
Tomorrow |
13-11-2017 |
The day after tomorrow |
14-11-2017 |
Three days from now |
15-11-2017 |
Four days later |
16-11-2017 |
Seventeenth of November |
17-11-2017 |
This Friday |
17-11-2017 |
Next Friday |
24-11-2017 |
Christmas Eve |
24-12-2017 |
A week before Christmas Eve |
17-12-2017 |
A year from now |
12-11-2018 |
Friday of the second week of January |
Query too complicated, date defaults to today |
123456789 |
Invalid date error shown |
!@#$%^&*() |
Invalid date error shown |
The parcel list is maintained in sorted order by comparing their delivery dates, with the earliest on top.
Status Field: Delivery Status of Parcel
Status
is used to indicate the current delivery status of a parcel. A parcel can have 4 possible delivery status and
listed below is a description of these Status
values.
Status | Description |
---|---|
PENDING |
This means that the parcel has not been delivered and has not passed the date it is supposed to be delivered by. |
DELIVERING |
This means that the parcel is currently working being delivered to its destination address. |
COMPLETED |
This indicates that the parcel has been successfully delivered to its destination. |
OVERDUE |
This state indicates that the parcel has not been delivered and has passed its due date. |
To input a Status
, you can type case-insensitive formats of the above Status. e.g. pending
or Pending
are valid
inputs to add a PENDING
Status
.
Values other than the above values will be rejected. e.g. PROCESSING , PROCESSED ;
|
Tags Field: Delivery Tags of Parcel
Tags
are used to indicate how the parcel should be handled. Tags can contain one or more of the following Tag
:
Tag | Description |
---|---|
FROZEN |
This means the parcel should be refrigerated as its contents are temperature sensitive. |
FLAMMABLE |
This means that the parcels' contents are highly flammable and should be kept away from heat. |
HEAVY |
This indicates that the parcel is heavy and may require additional manpower to deliver. |
FRAGILE |
This state indicates that the parcels' contents can be broken easily and requires additional care when handling. |
To input a Tag
, you can type insensitive formats of any of the above Tags
. e.g. frozen
or Frozen
are valid inputs
to add a FROZEN
Tag
.
Values other than the above values will be rejected. e.g. friends , colleagues .
If your use of Ark requires more tags to be made available, please contact our team and we will see to your request.
|
End of Extract
What is Ark?
Ark is a Command Line Interface software that helps delivery and shipping vendors manage their parcels and deliveries. Ark allows these delivery companies to track the parcels that they have to deliver to their customers, and empowers delivery vendors with simple end-to-end management of these deliveries. Ark also provides route optimization features to help vendors optimize their deliveries based on time or distance.
About this guide
The purpose of this developer guide is to help incoming developers, project managers and executives understand the
high-level details of the Ark software and help them develop code in coherence with our guidelines. Such details
include the Ark architecture and system, its components and the interaction between these components.
This guide contains information pertaining to issues such as:
-
Setting up the development environment for Ark.
-
Understanding the high-level components of Ark.
-
Understanding the various features and their implementations in Ark.
-
Adding documentation to document the changes you have made.
-
Running tests for the code in Ark.
-
Details on how to manage the Ark project using Version Control and Continuous Integration.
End of Extract
Here are some enhancements that I have proposed for my team’s project:
Enhancement Proposed: Clustering of Parcel Deliveries
Cluster parcels with Delivering
Status according to their address locations to ensure that deliveries in a single
cluster are delivered together.
Other contributions
-
Made the decision for the team to model the application’s GUI to be similar to that of iTunes
-
Main software developer for the team (you can view a summary of my contributions the github project page here
-
Refactored all instance of Person to Parcel in the codebase. You can view my pull request here here
-
Added Tooltip on mouseover of fields in the parcel. This was a necessary change since parts of the field will be missing and be replaced if with ellipsis
…
if it was too long. You can view my pull-request here -
Change name and icon of Ark. You can view the pull request here
-
Added Travis CI and coveralls to the Ark repository for automated deployment and testing.
-
Made Ark application open in list mode on startup. You can view the pull request here
-
Add end-to-end internal logic for parcel tab click (this change is pretty huge). You can view the pull request here
-
Fixed Acceptance Testing bugs for my team. Some examples:
-
Helped other teams do acceptance testing. Some examples:
-
Participate in forum. Some examples:
Other Project: NUSVisions
NUSVisions was an application I built over the Summer of 2017. NUSVisions is an application meant to help NUS students plan for the entire 4 years of their studies. It stores your sessions to ensure that modules saved in your plans remain after a period of time. I deployed the web application on a Heroku server and you can access it by clicking here