Project: Ark
Ark is a desktop delivery management application that my team and I had built for our Software Engineering module in the National University of Singapore. We built this application for delivery companies to manage their deliveries. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 14kLoC. You may access the Ark repository here.
Ark was built on top of Addressbook - Level 4, an addressbook application. A number of features were added and refactoring was done to develop the original codebase into the parcel management application, Ark.
You may access the links below for a list of more significant contributions I have made to Ark. Functional code refers to the code that I have written to enhance the Ark application while Test code refers to the code that I have written to automate testing for the Ark application.
Code contributed: [Functional code][Test code][Unused code]
Due Date Field: Due Date for Parcel Delivery
Delivery Date
is used to indicate the delivery date that the parcel must be delivered by.
The dates are only accepted if they are in the valid format DD-MM-YYYY or understandable by Ark.
Ark is able to recognise various forms of dates as shown in the table below but the dates in the Ark are formatted as DD-MM-YYYY. However, invalid inputs such as a phone number or symbols still will be rejected.
Current date as of writing is 12 November 2017.
User input | Date parsed by Ark |
---|---|
01-01-2017 |
01-01-2017 |
01/01/2017 |
01-01-2017 |
01.01.2017 |
01-01-2017 |
01-01-17 |
01-01-2017 |
First day of 2017 |
01-01-2017 |
The day before yesterday |
10-11-2017 |
Yesterday |
11-11-2017 |
Today |
12-11-2017 |
Tomorrow |
13-11-2017 |
The day after tomorrow |
14-11-2017 |
Three days from now |
15-11-2017 |
Four days later |
16-11-2017 |
Seventeenth of November |
17-11-2017 |
This Friday |
17-11-2017 |
Next Friday |
24-11-2017 |
Christmas Eve |
24-12-2017 |
A week before Christmas Eve |
17-12-2017 |
A year from now |
12-11-2018 |
Friday of the second week of January |
Query too complicated, date defaults to today |
123456789 |
Invalid date error shown |
!@#$%^&*() |
Invalid date error shown |
The parcel list is maintained in sorted order by comparing their delivery dates, with the earliest on top.
Justification
The delivery dates field is important as the list of parcels are sorted by delivery date with the earliest at the top. This allows for the user to look at the more pertinent deliveries immediately on start up.
Delivery Date field
Delivery Date
is used to indicate the delivery date that the parcel must be delivered by.
The dates are only accepted if they are in the valid format DD-MM-YYYY or understandable by Ark.
Ark is able to recognise various forms of dates as shown in the table below but the dates in the Ark are formatted as DD-MM-YYYY. However, invalid inputs such as a phone number or symbols still will be rejected.
Current date as of writing is 12 November 2017.
User input | Date parsed by Ark |
---|---|
01-01-2017 |
01-01-2017 |
01/01/2017 |
01-01-2017 |
01.01.2017 |
01-01-2017 |
01-01-17 |
01-01-2017 |
First day of 2017 |
01-01-2017 |
The day before yesterday |
10-11-2017 |
Yesterday |
11-11-2017 |
Today |
12-11-2017 |
Tomorrow |
13-11-2017 |
The day after tomorrow |
14-11-2017 |
Three days from now |
15-11-2017 |
Four days later |
16-11-2017 |
Seventeenth of November |
17-11-2017 |
This Friday |
17-11-2017 |
Next Friday |
24-11-2017 |
Christmas Eve |
24-12-2017 |
A week before Christmas Eve |
17-12-2017 |
A year from now |
12-11-2018 |
Friday of the second week of January |
Query too complicated, date defaults to today |
123456789 |
Invalid date error shown |
!@#$%^&*() |
Invalid date error shown |
The parcel list is maintained in sorted order by comparing their delivery dates, with the earliest on top.
The following sequence diagram shows how the delivery date is parsed to PrettyTime's parser:
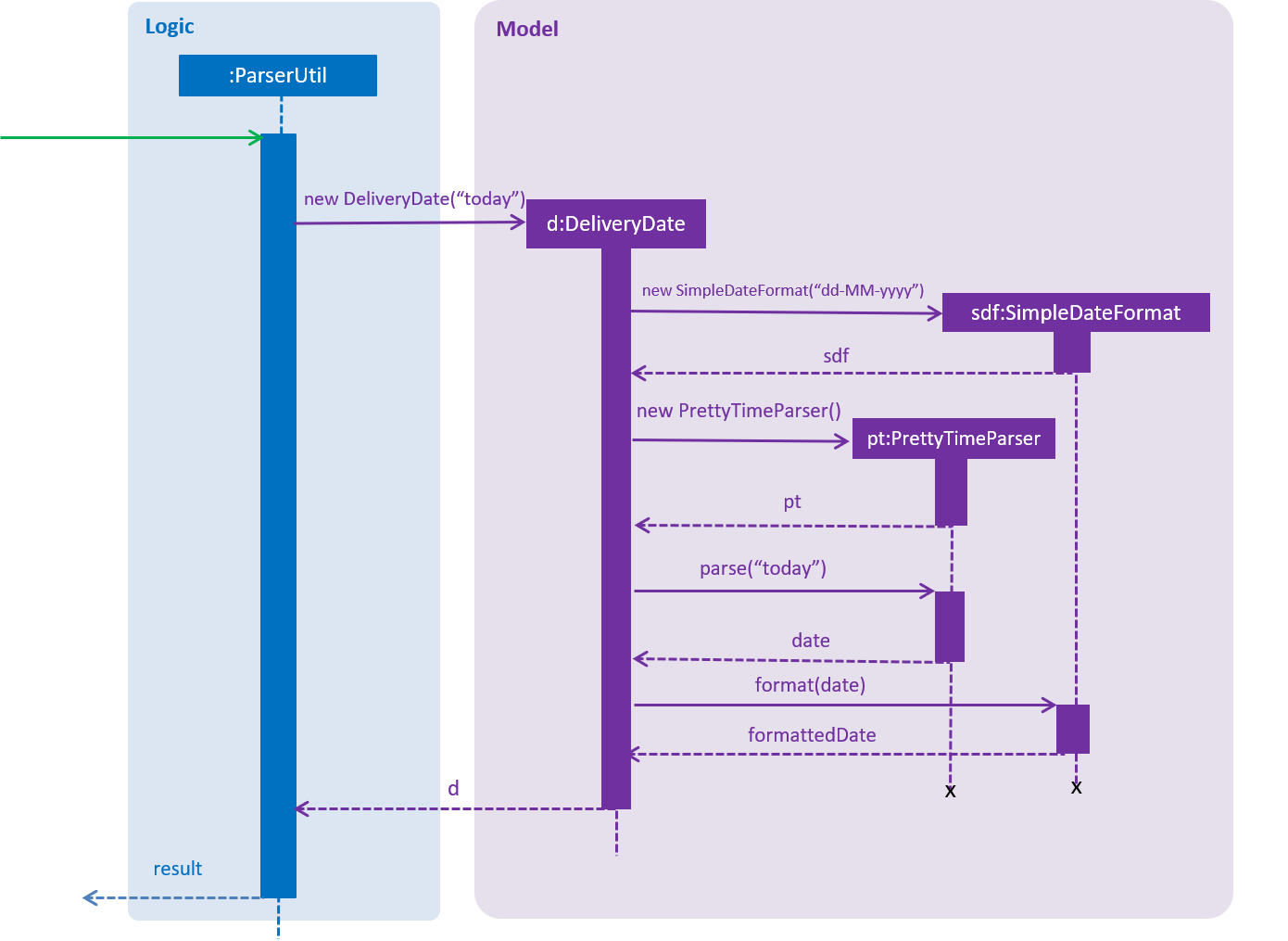
Figure 4.13.1 : Sequence diagram describing the parsing of delivery date when edit 1 d/today
is executed
As seen in the sequence diagram above, a request comes in to ParserUtil to parse the delivery date. A new
DeliveryDate is then created and within it we instantiate a SimpleDateFormat object with the desired date
format and an instance of PrettyTimeParser. We then request for PrettyTimeParser to parse the string today
and subsequently pass it’s result into our SimpleDateFormat to format our date in the way we’ve defined, dd-MM-yyyy
.
After the date has been formatted, we store it within the DeliveryDate object to be returned to ParserUtil and
subsequently returned to whichever method which called it.
Maintaining a Sorted List
The list of parcels stored in Ark is maintained to be sorted according to the delivery date of
the parcels, with the earliest delivery date at the top. This allows the more pertinent
deliveries to be shown quickly.
The list is sorted whenever there is a change in the list that may potentially disrupt the order
of the list so that the user does not have to manually do so.
Justification
Having the list in sorted order allows the user to look at the more pertinent deliveries more easily.
Maintain sorted mechanism
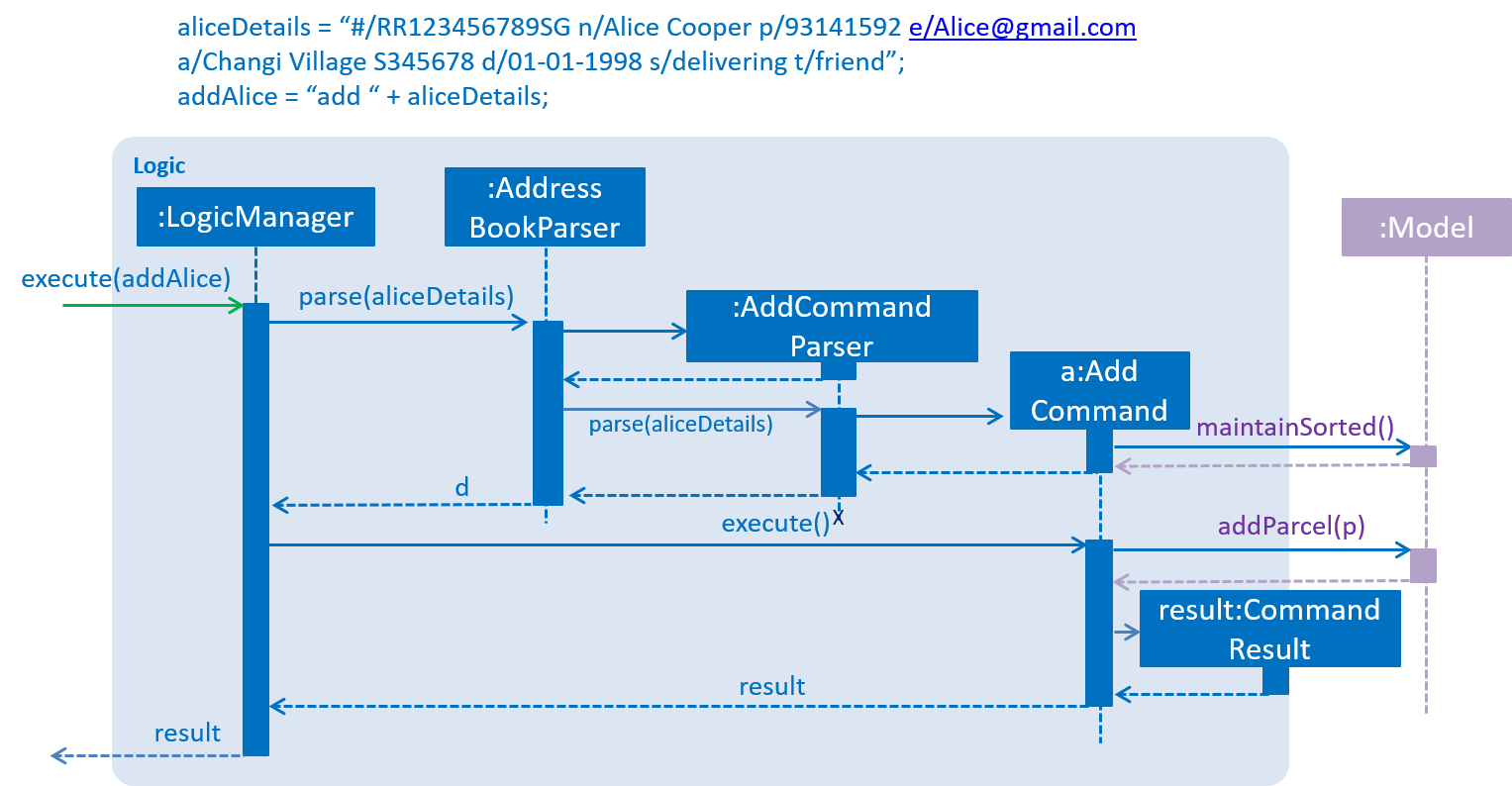
Figure 4.15.1 : Adding Alice to Ark, maintainSorted is actually called and returns void.
The list of parcels in Ark is maintained to be always in sorted order according to delivery dates,
with the earliest being on the top. This is so that the user will be able to look at the more
pertinent deliveries.
The list is sorted whenever a parcel is added or edited. This is
because these commands are the ones that might possibly cause the new parcel to be placed
in the wrong position.
Ark has two tabs, one for uncompleted parcels, the other for completed parcels. Whenever add
and edit
commands
are executed, the newly added or edited parcel gets selected. If the status of the newly added or edited parcel is
not on the current active list, a tab switch needs to happen. This is taken care off by the selection mechanism within
the Model Manager class.
Design Considerations
Aspect: Implementation of maintainSorted
-
Alternative 1 (current choice): Constant sort the list of parcels whenever there is a change that potentially could disrupt the order of the list.
-
Pros: Intuitive and guarantees that list is sorted in the right order
-
Cons: Many commands have to be changed
-
-
Alternative 2: Insert the new / edited parcel to fit into the sorted list.
-
Pros: Use less computation as the list of parcels is already sorted.
-
Cons: More difficult to implement as we’ll need to implement our own sorting algorithm as opposed to just using the built in sorting methods.
-
ArkBot: Telegram Bot Interface for Ark
An Overview
As a desktop application, isn’t it rather cumbersome for a delivery man to check and update information on the go, when he is making his deliveries? We thought so to. As such we developed a Telegram Bot Interface for Ark so that the delivery man would be able to conveniently see which deliveries he has remaining and their addresses. Furthermore, he will be able to mark the deliveries as complete easily from the convenience of his phone.
Like all Telegram Bots, each command must be prefixed with a /
character. So if I were to want to trigger the help
command, I would send /help
to ArkBot. The command formats are identical to that of Ark to make things easy to recall.
Configuring ArkBot
Telegram Bots require an Authorization Token which make them unique. To make the ArkBot respond only to your Ark software, head here to learn how to retrieve an Authorization Token from Telegram’s Botfather.
The following steps illustrate how you should retrieve and configure the Authorization Token:
-
Retrieve Authorization Token from Botfather.
-
Your token should look something like this:
458231237:AAEPeghjklUxY7GHnYiJ5c0y-IldALLI0FE
-
-
Initialize [T16-B1][Ark].jar to generate the default configuration file, config.json.
-
Open config.json with your favourite text editor.
-
Some common text editors include Notepad or Vim.
-
-
Change the value of the
botToken
field as shown in Figure 6.19.2.1. -
Restart Ark and you should be all set!
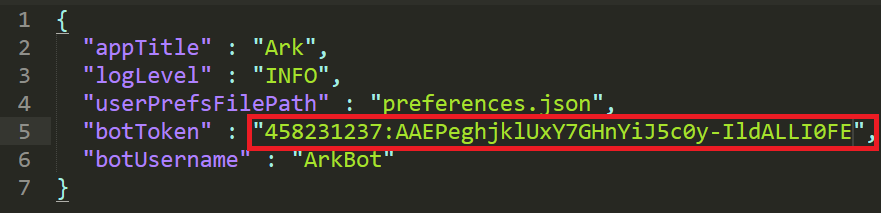
Figure 6.19.2.1 : Sample Configuration File with Telegram Bot Authorization Token field highlighted.
After which, enter the authorization token into the config.json file that is created in the same folder you placed the Ark software executable file. This token is especially important as anyone with access to it will be able to access the information that is sent and received by the bot.
ArkBot Commands
Here the commands that are available in ArkBot.
Command | Description |
---|---|
/all #/TRACKING_NUMBER n/NAME [p/PHONE_NUMBER] [e/EMAIL] a/ADDRESS d/DELIVERYDATE [s/STATUS] [t/TAG]…` |
Adds a parcel. |
/list |
Lists uncompleted parcel deliveries. |
/find KEYWORD [MORE_KEYWORDS] |
List uncompleted parcels with keywords in names. |
/delete [Parcel Index] |
Deletes a parcel. |
/undo |
Undo a command. |
/redo |
Redo a command. |
/complete [Parcel Index] |
Marks a parcel as completed. |
/complete |
Activates listen mode. |
/cancel |
Cancels listen mode. |
/help |
Brings up help dialogue with information on commands. |
ArkBot Listen Mode
If /complete
is entered without a parcel index, ArkBot will enter listen mode
. In listen mode
, ArkBot will wait
for an image QR code to be sent. The QR code must contain details of the parcel and the parcel must be present in the
Ark’s list of parcels.
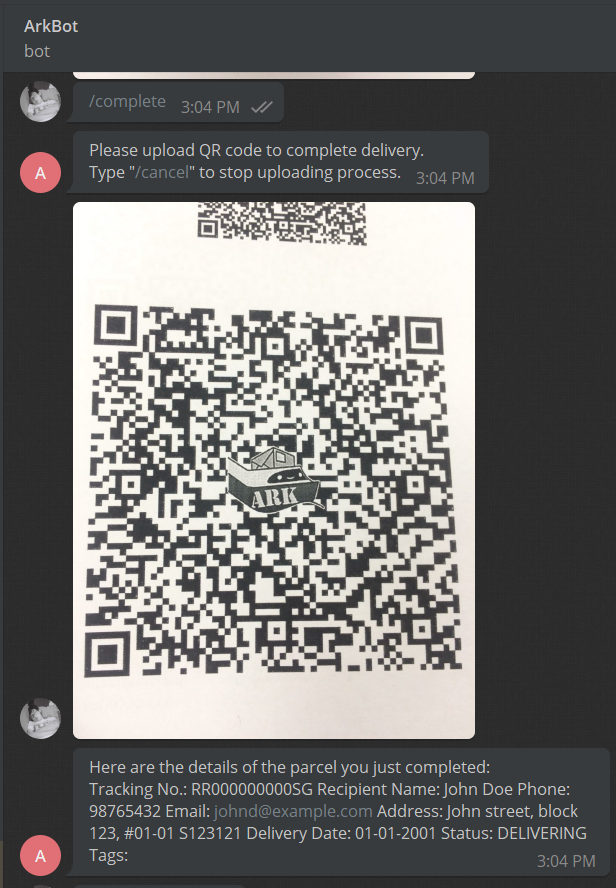
Figure 6.19.3.1: Sample interaction of sending QR code to ArkBot
As shown in Figure 6.21.3.1, upon typing /complete
, ArkBot will prompt the user for an image. If ArkBot is able to
recognise the parcel, it will reply with the parcel’s previous details as shown and mark it as completed on Ark.
If ArkBot is not in listen mode
and a QR code with parcel details are sent, ArkBot assumes that the user is trying to
read the information on the QR code and displays it.
Justification
Practically speaking, a delivery man would not be able to use Ark on the go since it is a desktop application. As such, I developed a Telegram Bot interface, enabling Ark to be accessed from a mobile phone.
ArkBot: Telegram Bot Interface for Ark
ArkBot is written using TelegramBots, a Java library written by rubenlagus to create bots using Telegram Bots API.
At present the following commands have an equivalent in ArkBot: add
delete
list
find
undo
redo
help
. ArkBot also has the added functionality of complete
which is merely a wrapper around the edit
command to mark parcel
deliveries as complete. This is especially useful for our delivery man.
Like all Telegram Bots, each command must be prefixed with a /
character. So if I were to want to trigger the help
command, I would send /help
to ArkBot.
At present, all messages and commands to ArkBot are sent directly to Ark where they are processed and the commands and arguments are formatted as Ark commands and subsequently executed.
If we enter the complete
command into ArkBot without any parameters, we enter listen
mode. In listen
mode, ArkBot is waiting for a QR to be sent to be analysed. The image is then downloaded and using the
zxing barcode scanning library for Java, the information is unwrapped and parsed into
the edit command to change the status of the parcel to COMPLETED
.
Design Considerations
Aspect: Implementation of ArkBot
-
Alternative 1 (current choice): Parse the arguments from the user into various commands as needed. If there any errors that surface, reply user with default error message.
-
Pros: Functions of commands on Ark remain the same, reusing current implementation
-
Cons: Error catching is difficult, it is difficult to test and slower as information is handle by many parties.
-
-
Alternative 2: Program each command from the Bot to directly interface with Model and Logic Managers on Ark
-
Pros: Quicker and more director way of communication. Less reliant on Ark commands.
-
Cons: More difficult to implement as we’ll need to implement our own version of all the command on Ark.
-
Project: GooBot
Over the summer of 2017, my partner and I developed a Telegram Bot generating framework using Node.js. Our framework allows users without programming experience to create their own Finite State Machine style Telegram bots. I specifically worked on data manipulation and created a simple HTML to allow users to manage their bots.
Goobot, which was created during the NUS Orbital Programme, won 1st prize in Gemini (Intermediate) category during the course’s project showcase.