By: CS2103AUG2017 Team T16-B1
Since: Sept 2017
Licence: MIT
- 1. Introduction
- 2. Setting up
- 3. Design
- 4. Implementation
- 4.1. Undo/Redo mechanism
- 4.2. Logging
- 4.3. Configuration
- 4.4. Google Maps Search Browser
- 4.5. Tab change mechanism
- 4.6. Overdue parcels popup window
- 4.7. Backup Mechanism
- 4.8. Import Mechanism
- 4.9. Tab autocomplete mechanism
- 4.10. Tracking Number Field
- 4.11. Postal Code Field
- 4.12. Status field
- 4.13. Delivery Date field
- 4.14. Tags Field
- 4.15. Maintain sorted mechanism
- 4.16. ArkBot: Telegram Bot Interface for Ark
- 5. Documentation
- 6. Testing
- 7. Dev Ops
- 8. Known Bugs
- Appendix A: User Stories
- Appendix B: Use Cases
- Appendix C: Non Functional Requirements
- Appendix D: Glossary
- Appendix E: Product Survey
1. Introduction
1.1. What is Ark?
Ark is a Command Line Interface software that helps delivery and shipping vendors manage their parcels and deliveries. Ark allows these delivery companies to track the parcels that they have to deliver to their customers, and empowers delivery vendors with simple end-to-end management of these deliveries. Ark also provides route optimization features to help vendors optimize their deliveries based on time or distance.
1.2. About this guide
The purpose of this developer guide is to help incoming developers, project managers and executives understand the
high-level details of the Ark software and help them develop code in coherence with our guidelines. Such details
include the Ark architecture and system, its components and the interaction between these components.
This guide contains information pertaining to issues such as:
-
Setting up the development environment for Ark.
-
Understanding the high-level components of Ark.
-
Understanding the various features and their implementations in Ark.
-
Adding documentation to document the changes you have made.
-
Running tests for the code in Ark.
-
Details on how to manage the Ark project using Version Control and Continuous Integration.
2. Setting up
2.1. Prerequisites
-
JDK
1.8.0_60
or laterHaving any Java 8 version is not enough.
This app will not work with earlier versions of Java 8. -
IntelliJ IDE
IntelliJ by default has Gradle and JavaFx plugins installed.
Do not disable them. If you have disabled them, go toFile
>Settings
>Plugins
to re-enable them.
2.2. Setting up the project in your computer
-
Fork this repo, and clone the fork to your computer
-
Open IntelliJ (if you are not in the welcome screen, click
File
>Close Project
to close the existing project dialog first) -
Set up the correct JDK version for Gradle
-
Click
Configure
>Project Defaults
>Project Structure
-
Click
New…
and find the directory of the JDK
-
-
Click
Import Project
-
Locate the
build.gradle
file and select it. ClickOK
-
Click
Open as Project
-
Click
OK
to accept the default settings -
Open a console and run the command
gradlew processResources
(Mac/Linux:./gradlew processResources
). It should finish with theBUILD SUCCESSFUL
message.
This will generate all resources required by the application and tests.
2.3. Verifying the setup
-
Run the
seedu.address.MainApp
and try a few commands -
Run the tests to ensure they all pass.
2.4. Configurations to do before writing code
2.4.1. Configuring the coding style
This project follows oss-generic coding standards. IntelliJ’s default style is mostly compliant with ours but it uses a different import order from ours. To rectify,
-
Go to
File
>Settings…
(Windows/Linux), orIntelliJ IDEA
>Preferences…
(macOS) -
Select
Editor
>Code Style
>Java
-
Click on the
Imports
tab to set the order-
For
Class count to use import with '*'
andNames count to use static import with '*'
: Set to999
to prevent IntelliJ from contracting the import statements -
For
Import Layout
: The order isimport static all other imports
,import java.*
,import javax.*
,import org.*
,import com.*
,import all other imports
. Add a<blank line>
between eachimport
-
Optionally, you can follow the UsingCheckstyle.adoc document to configure Intellij to check style-compliance as you write code.
2.4.2. Updating documentation to match your fork
After forking the repo, links in the documentation will still point to the CS2103AUG2017-T16-B1/main
repo. If you
plan to develop this as a separate product (i.e. instead of contributing to the CS2103AUG2017-T16-B1/main
) ,
you should replace the URL in the variable repoURL
in DeveloperGuide.adoc
and UserGuide.adoc
with the
URL of your fork.
2.4.3. Setting up CI
Set up Travis to perform Continuous Integration (CI) for your fork. See UsingTravis.adoc to learn how to set it up.
Optionally, you can set up AppVeyor as a second CI (see UsingAppVeyor.adoc).
Having both Travis and AppVeyor ensures your App works on both Unix-based platforms and Windows-based platforms (Travis is Unix-based and AppVeyor is Windows-based) |
2.4.4. Getting started with coding
When you are ready to start coding,
Before you start contributing to Ark, get some sense of the overall design by reading the Architecture section.
3. Design
3.1. Architecture
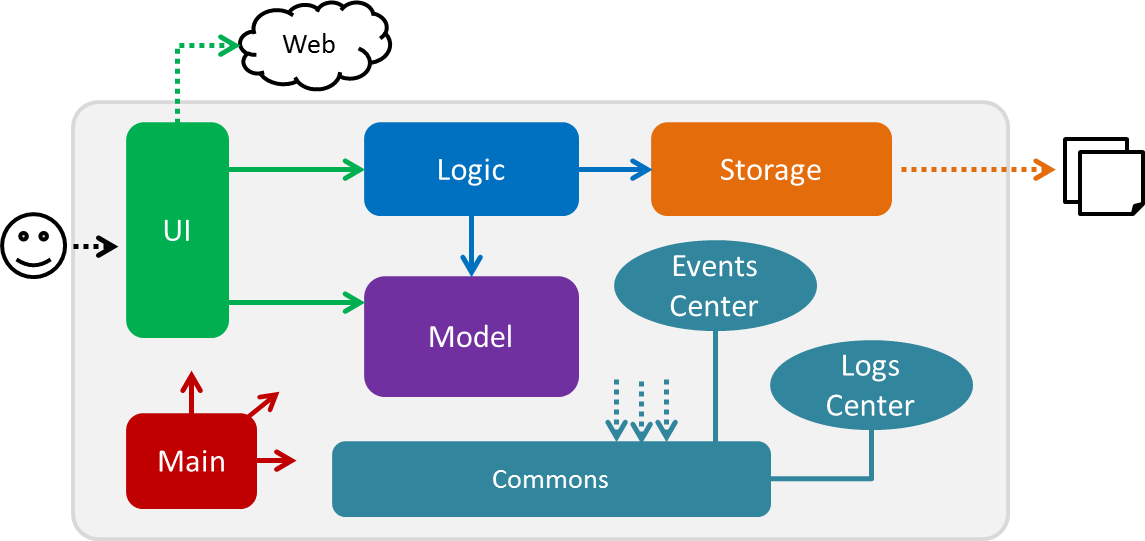
Figure 3.1.1 : Architecture Diagram
The Architecture Diagram given above explains the high-level design of the App. Given below is a quick overview of each component.
The .pptx files used to create diagrams in this document can be found in the diagrams
folder. To update a diagram, modify the diagram in the pptx file, select the objects of the diagram, and choose Save
as picture .
|
Main
has only one class called MainApp
. It is responsible
for,
-
At app launch: Initializes the components in the correct sequence, and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
Commons
represents a collection of classes used by multiple other components. Two of those
classes play important roles at the architecture level.
-
EventsCenter
: This class (written using Google’s Event Bus library) is used by components to communicate with other components using events (i.e. a form of Event Driven design) -
LogsCenter
: Used by many classes to write log messages to the App’s log file.
The rest of the App consists of four components.
Each of the four components
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name}Manager
class.
For example, the Logic
component (see the class diagram given below) defines it’s API in the Logic.java
interface
and exposes its functionality using the LogicManager.java
class.
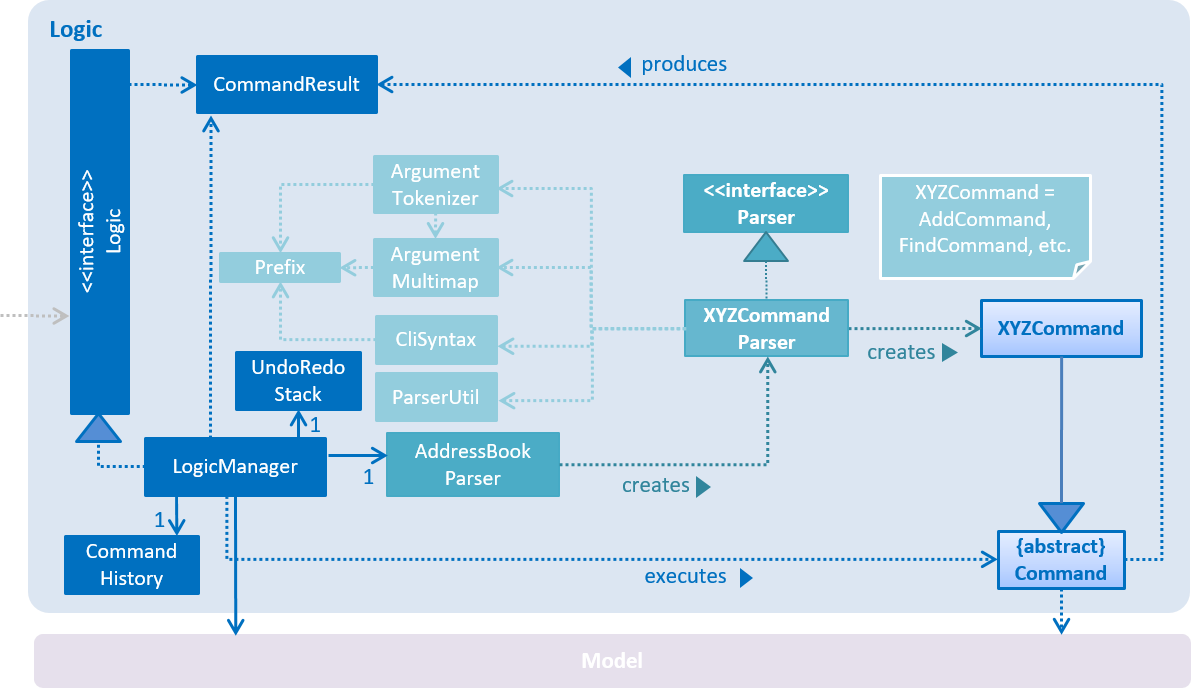
Figure 3.1.2 : Class Diagram of the Logic Component
Events-Driven nature of the design
The Sequence Diagram below shows how the components interact for the scenario where the user issues the command
delete 1
.

Figure 3.1.3a : Component interactions for delete 1
command (part 1)
Note how the Model simply raises a AddressBookChangedEvent when the address book data are changed, instead of
asking the Storage to save the updates to the hard disk.
|
The diagram below shows how the EventsCenter
reacts to that event, which eventually results in the updates being
saved to the hard disk and the status bar of the UI being updated to reflect the 'Last Updated' time.

Figure 3.1.3b : Component interactions for delete 1
command (part 2)
Note how the event is propagated through the EventsCenter to the Storage and UI without Model having to be
coupled to either of them. This is an example of how this Event Driven approach helps us reduce direct coupling between
components.
|
The sections below give more details of each component.
3.2. UI component
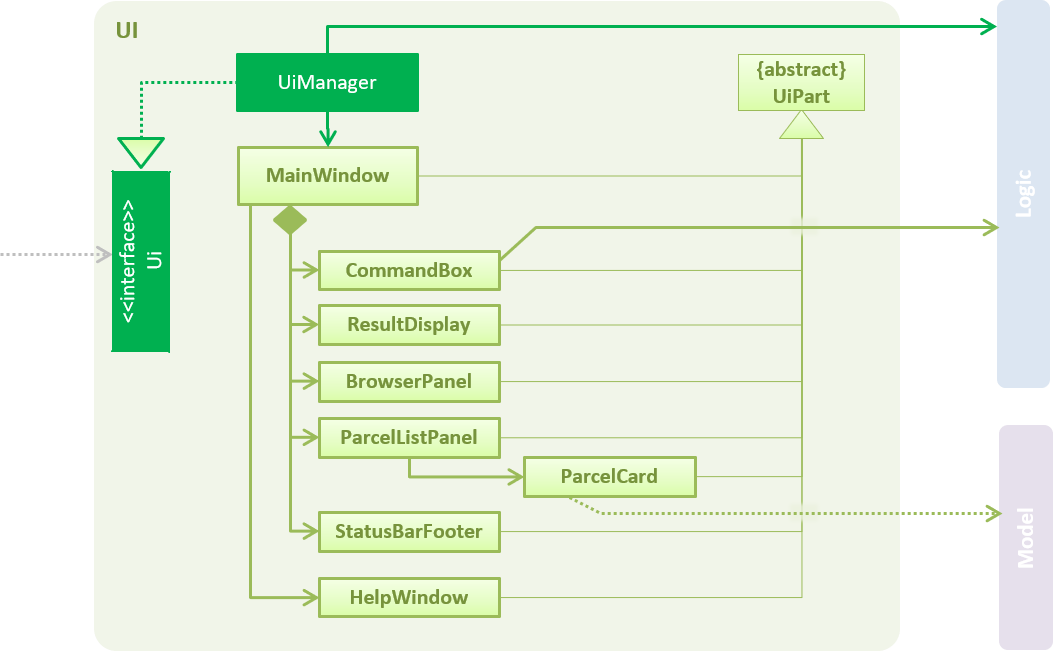
Figure 3.2.1 : Structure of the UI Component
API : Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, ParcelListPanel
,
StatusBarFooter
, BrowserPanel
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that
are in the src/main/resources/view
folder. For example, the layout of the
MainWindow
is specified in
MainWindow.fxml
The UI
component,
-
Executes user commands using the
Logic
component. -
Binds itself to some data in the
Model
so that the UI can auto-update when data in theModel
change. -
Responds to events raised from various parts of the App and updates the UI accordingly.
3.3. Logic component
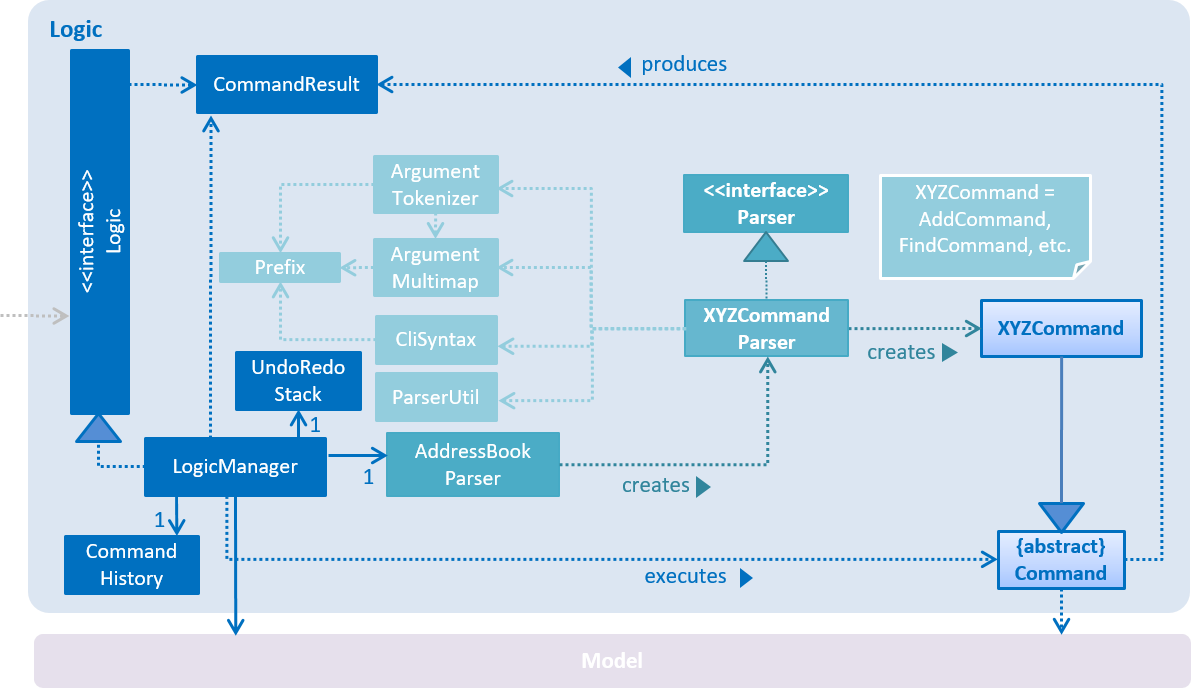
Figure 3.3.1 : Structure of the Logic Component
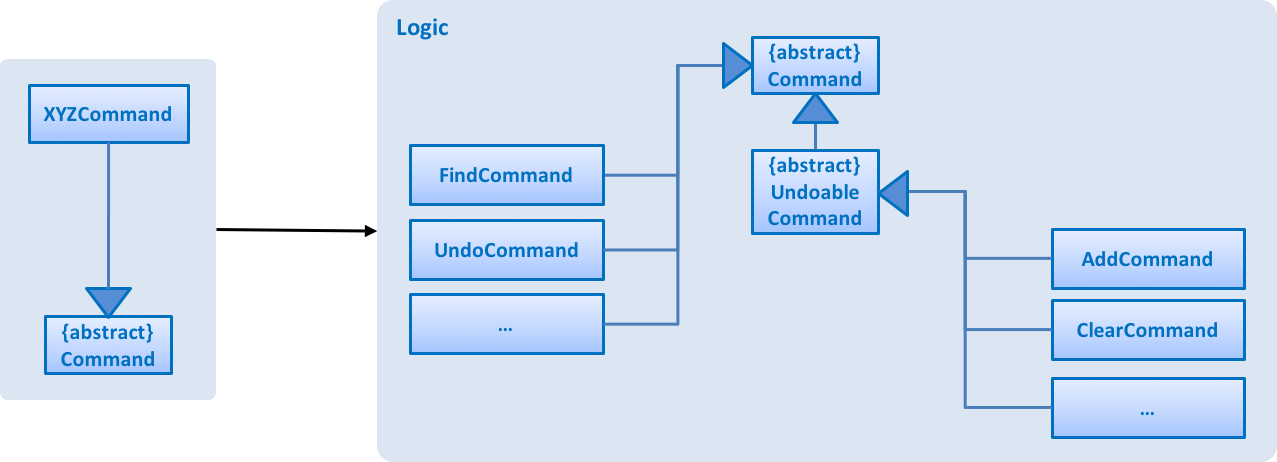
Figure 3.3.2 : Structure of Commands in the Logic Component. This diagram shows finer details concerning XYZCommand
and Command
in Figure 3.3.1
API :
Logic.java
-
Logic
uses theAddressBookParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding a parcel) and/or raise events. -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("delete 1")
API
call.
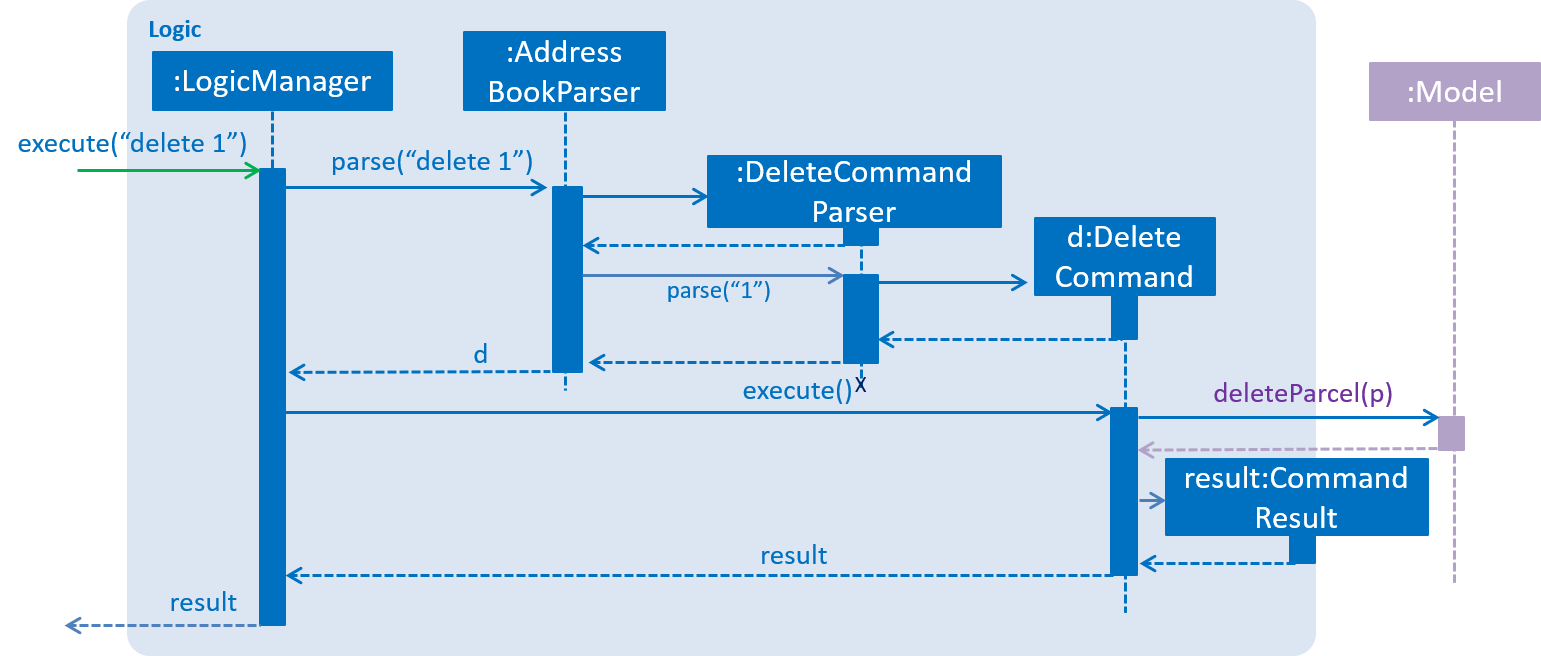
Figure 3.3.3 : Interactions Inside the Logic Component for the delete 1
Command
3.4. Model component
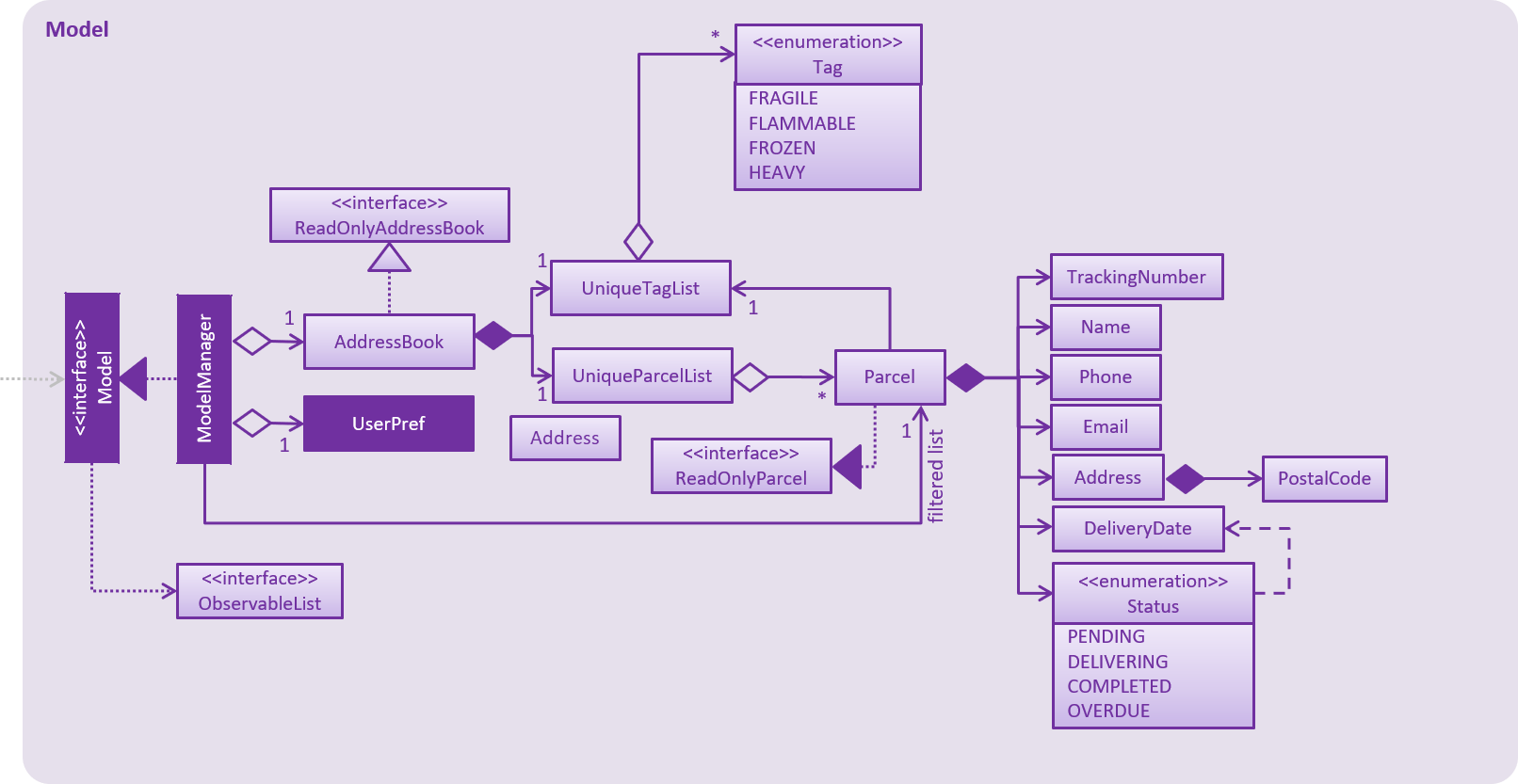
Figure 3.4.1 : Structure of the Model Component
API : Model.java
The Model
,
-
stores a
UserPref
object that represents the user’s preferences. -
stores the data from interactions with AddressBook.
-
exposes an unmodifiable
ObservableList<ReadOnlyParcel>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
does not depend on any of the other three components.
3.5. Storage component
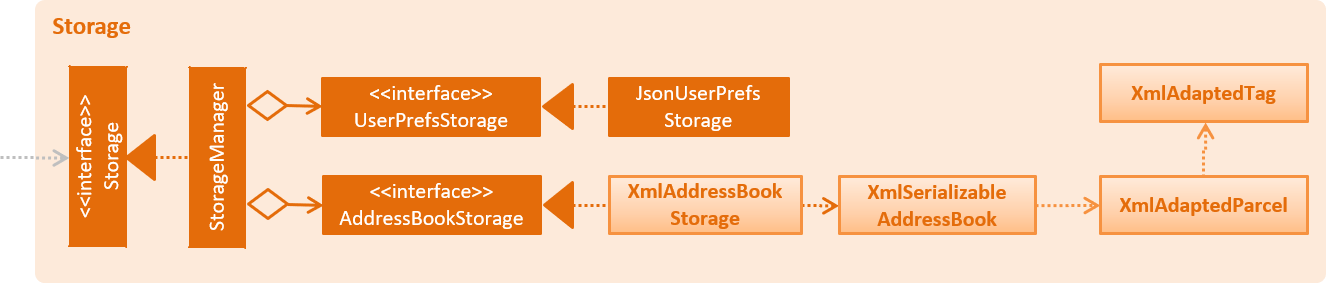
Figure 3.5.1 : Structure of the Storage Component
API : Storage.java
The Storage
component,
-
can save
UserPref
objects in json format and read it back. -
can save the Address Book data in xml format and read it back.
3.6. Common classes
Classes used by multiple components are in the seedu.addressbook.commons
package.
4. Implementation
This section describes some noteworthy details on how certain features are implemented.
4.1. Undo/Redo mechanism
The undo/redo mechanism is facilitated by an UndoRedoStack
, which resides inside LogicManager
. It supports undoing
and redoing of commands that modifies the state of address book (e.g. add
, edit
). Such commands will inherit from
UndoableCommand
.
UndoRedoStack
only deals with UndoableCommands
. Commands that cannot be undone will inherit from Command
instead.
The following diagram shows the inheritance diagram for commands:
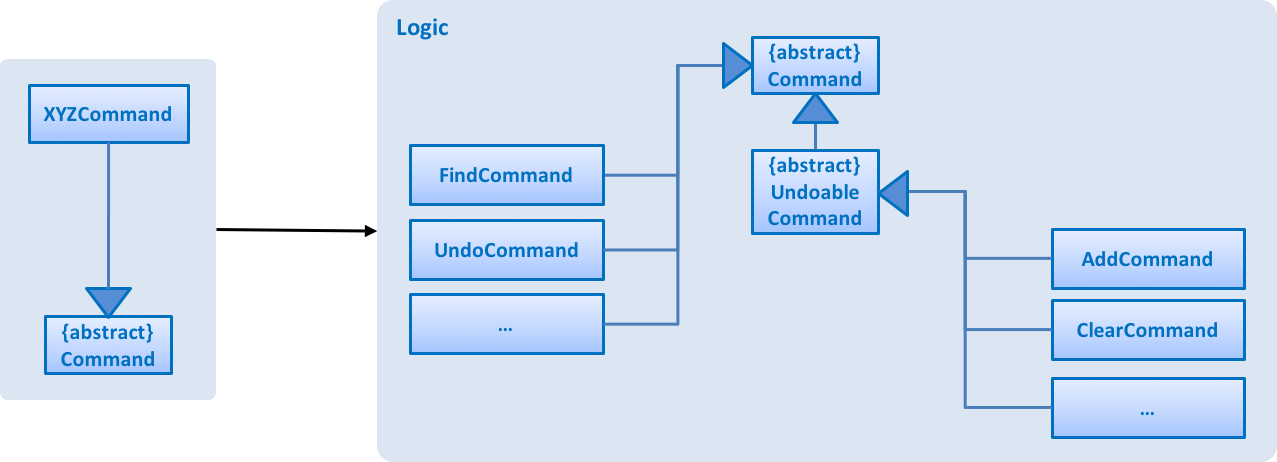
Figure 4.1.1 : Inheritance diagram for commands
As you can see from the diagram, UndoableCommand
adds an extra layer between the abstract Command
class and
concrete commands that can be undone, such as the DeleteCommand
. Note that extra tasks need to be done when executing
a command in an undoable way, such as saving the state of the address book before execution. UndoableCommand
contains the high-level algorithm for those extra tasks while the child classes implements the details of how to execute
the specific command. Note that this technique of putting the high-level algorithm in the parent class and lower-level
steps of the algorithm in child classes is also known as the
template pattern.
Commands that are not undoable are implemented this way:
public class ListCommand extends Command {
@Override
public CommandResult execute() {
// ... list logic ...
}
}
With the extra layer, the commands that are undoable are implemented this way:
public abstract class UndoableCommand extends Command {
@Override
public CommandResult execute() {
// ... undo logic ...
executeUndoableCommand();
}
}
public class DeleteCommand extends UndoableCommand {
@Override
public CommandResult executeUndoableCommand() {
// ... delete logic ...
}
}
Suppose that the user has just launched the application. The UndoRedoStack
will be empty at the beginning.
The user executes a new UndoableCommand
, delete 5
, to delete the 5th parcel in the address book. The current state
of the address book is saved before the delete 5
command executes. The delete 5
command will then be pushed onto
the undoStack
(the current state is saved together with the command).
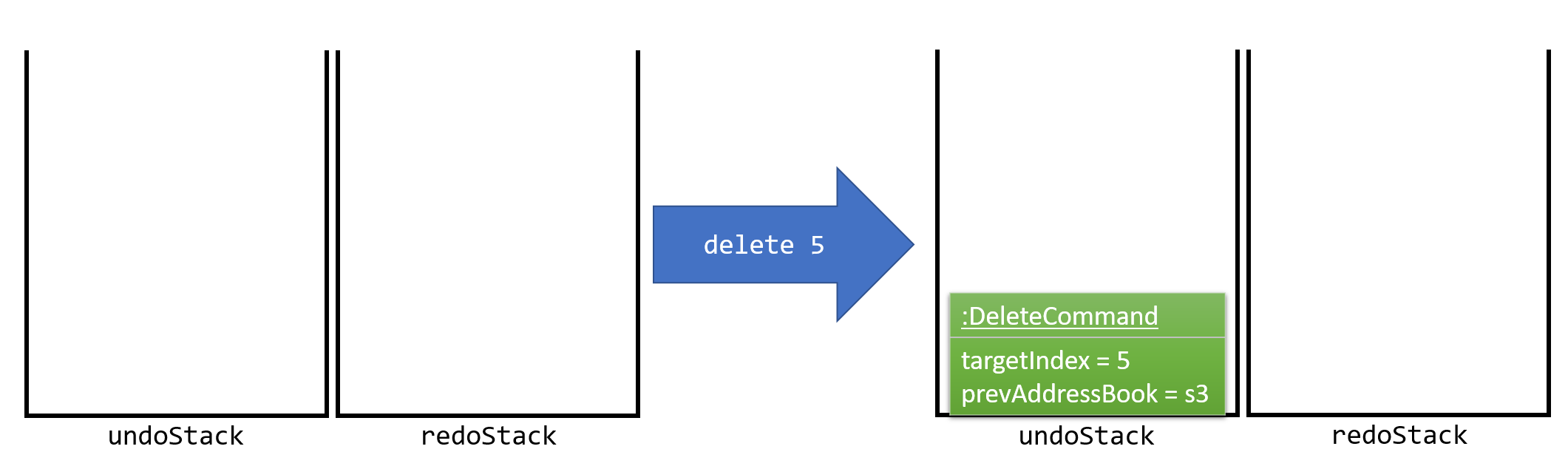
Figure 4.1.2 : State of the undoStack and redoStack after delete 5
is executed
As the user continues to use the program, more commands are added into the undoStack
. For example, the user may
execute add n/David …
to add a new parcel.
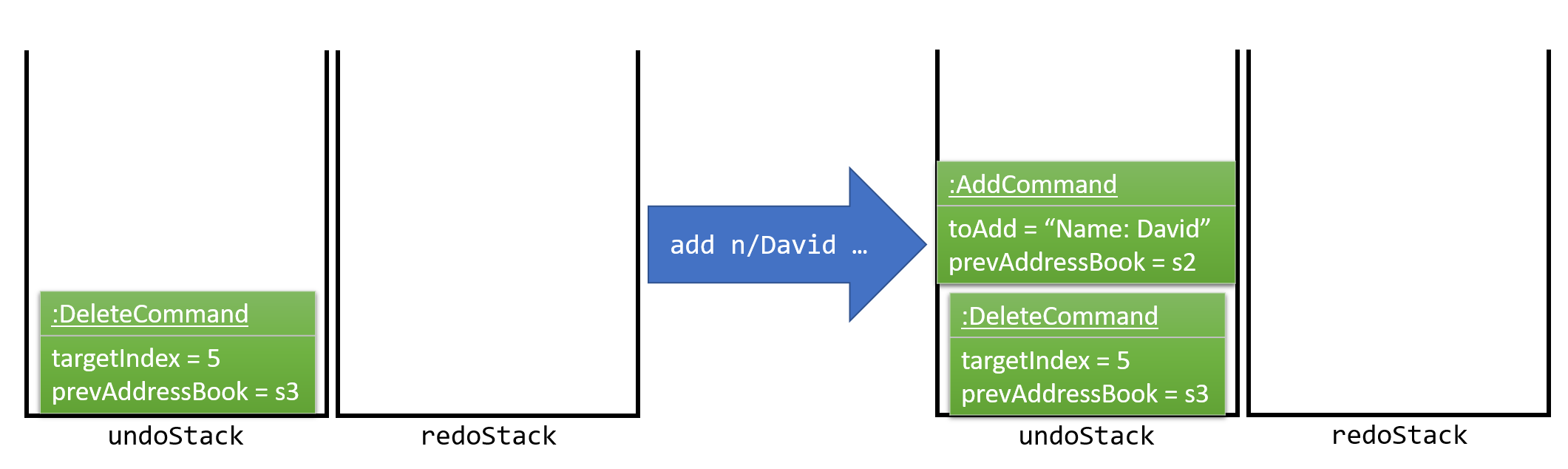
Figure 4.1.2 : State of the undoStack and redoStack after add n/David
is executed
If a command fails its execution, it will not be pushed to the UndoRedoStack at all.
|
The user now decides that adding the parcel was a mistake, and decides to undo that action using undo
.
We will pop the most recent command out of the undoStack
and push it back to the redoStack
. We will restore the
address book to the state before the add
command executed.
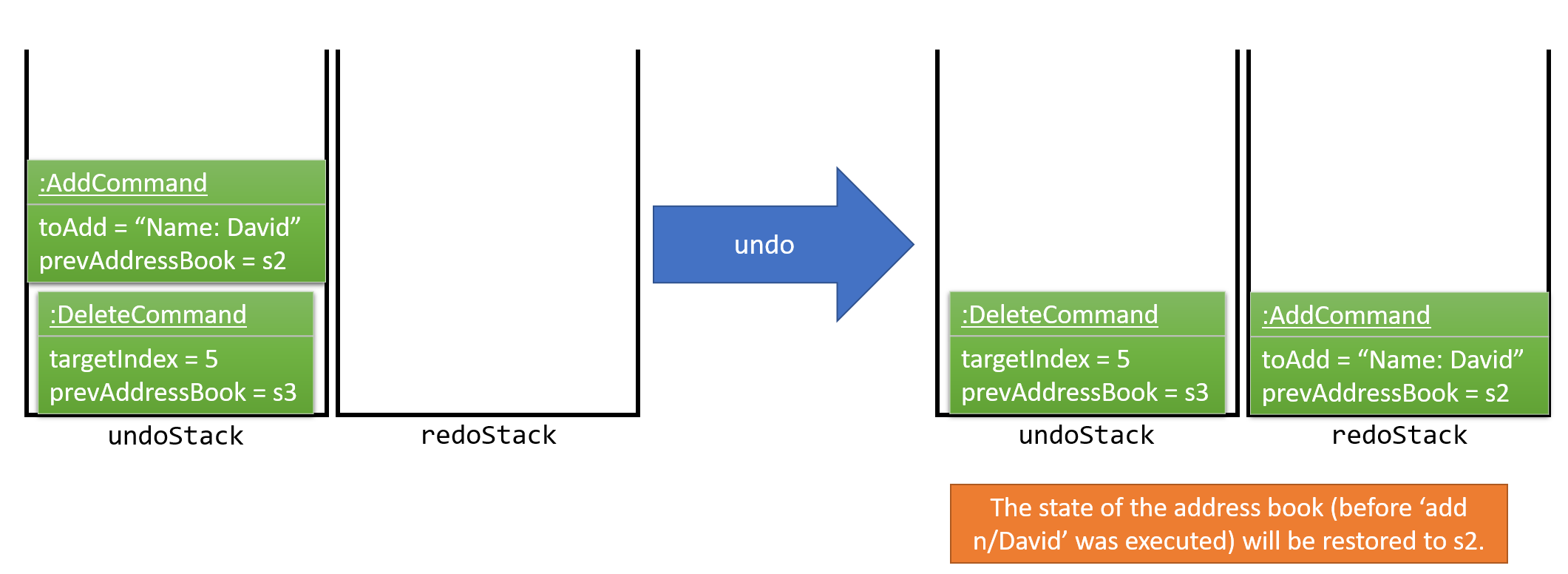
Figure 4.1.3 : State of the undoStack and redoStack after undo
is executed
If the undoStack is empty, then there are no other commands left to be undone, and an Exception will be thrown when
popping the undoStack .
|
The following sequence diagram shows how the undo operation works:
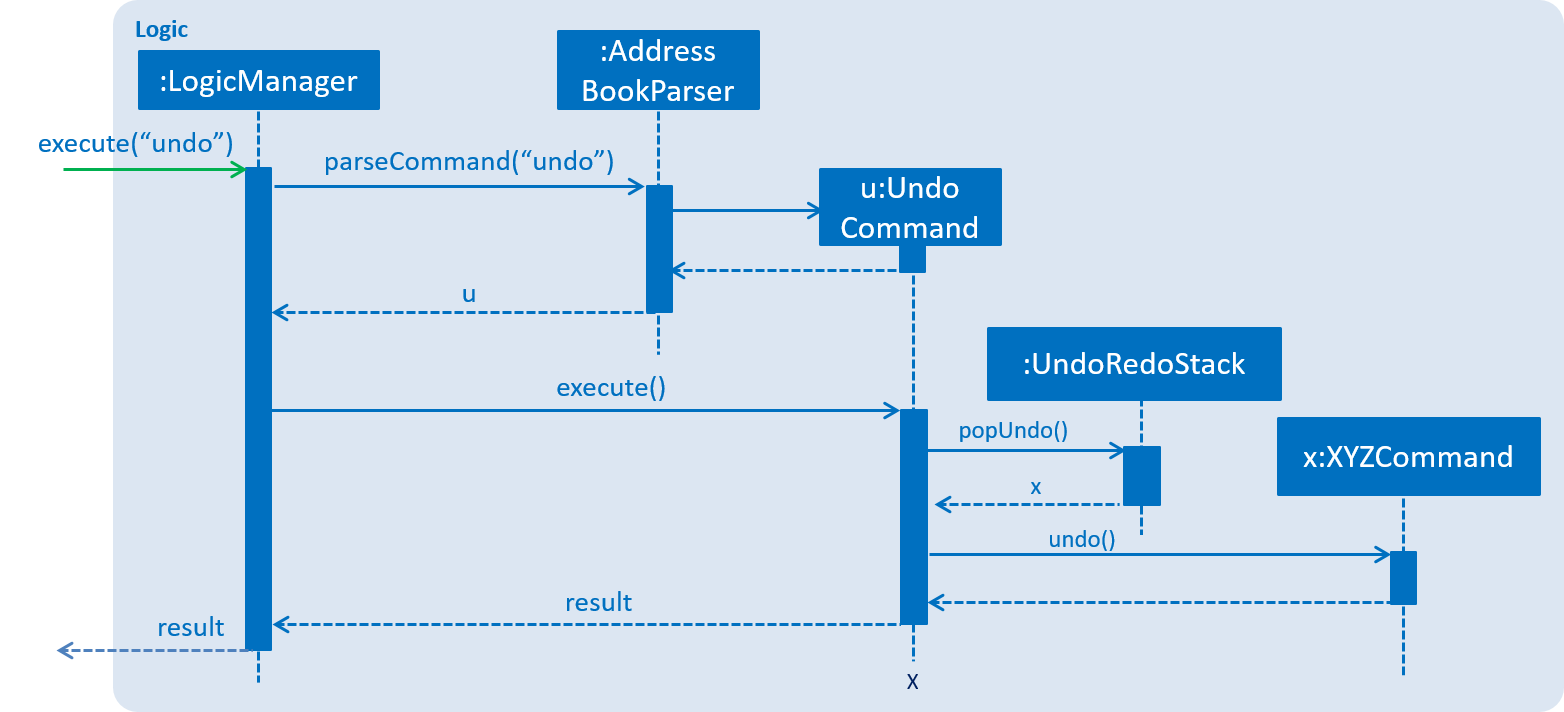
Figure 4.1.4 : Sequence diagram of the undo operation
The redo does the exact opposite (pops from redoStack
, push to undoStack
, and restores the address book to the
state after the command is executed).
If the redoStack is empty, then there are no other commands left to be redone, and an Exception will be thrown when
popping the redoStack .
|
The user now decides to execute a new command, clear
. As before, clear
will be pushed into the undoStack
. This
time the redoStack
is no longer empty. It will be purged as it no longer make sense to redo the add n/David
command
(this is the behavior that most modern desktop applications follow).
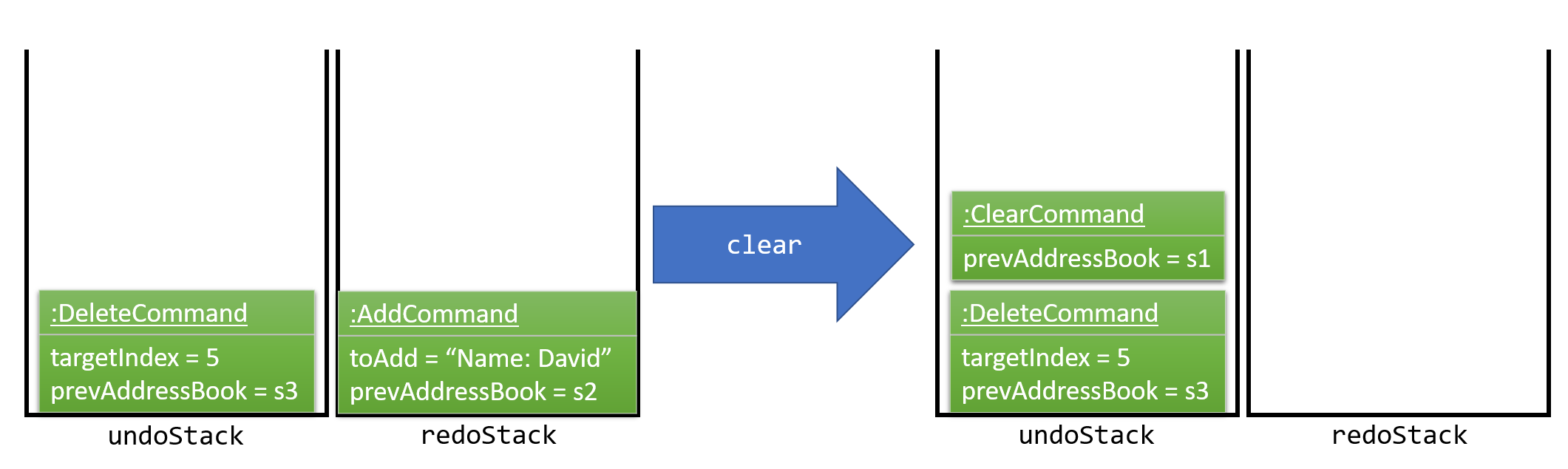
Figure 4.1.5 : State of the undoStack and redoStack after clear
is executed
Commands that are not undoable are not added into the undoStack
. For example, list
, which inherits from Command
rather than UndoableCommand
, will not be added after execution:
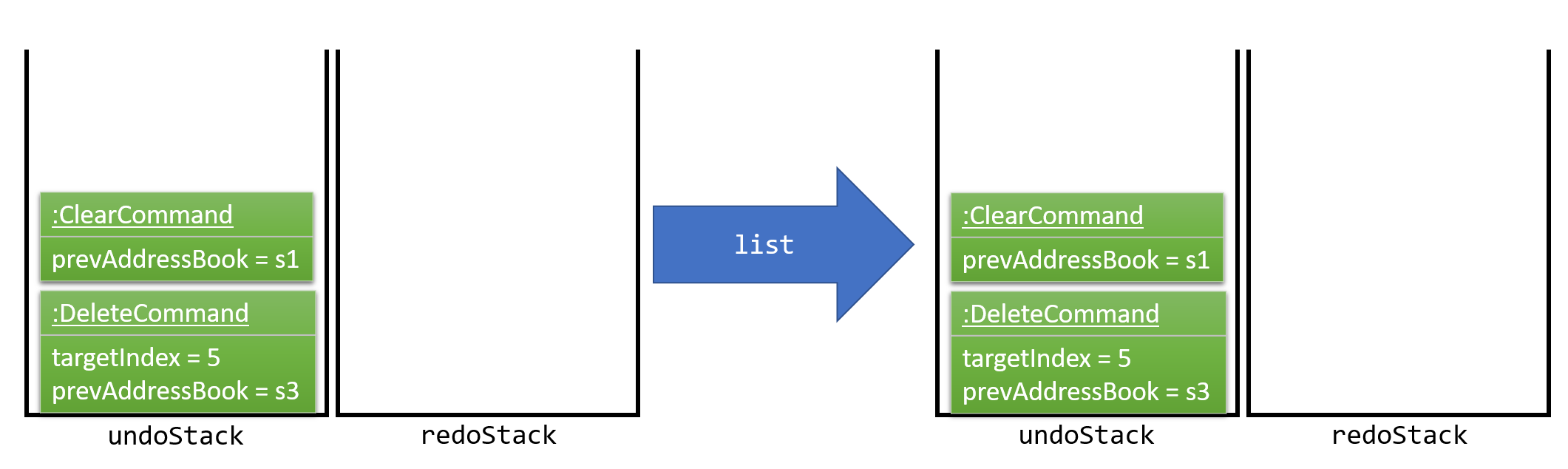
Figure 4.1.6 : State of the undoStack and redoStack after list
is executed
The following activity diagram summarize what happens inside the UndoRedoStack
when a user executes a new command:
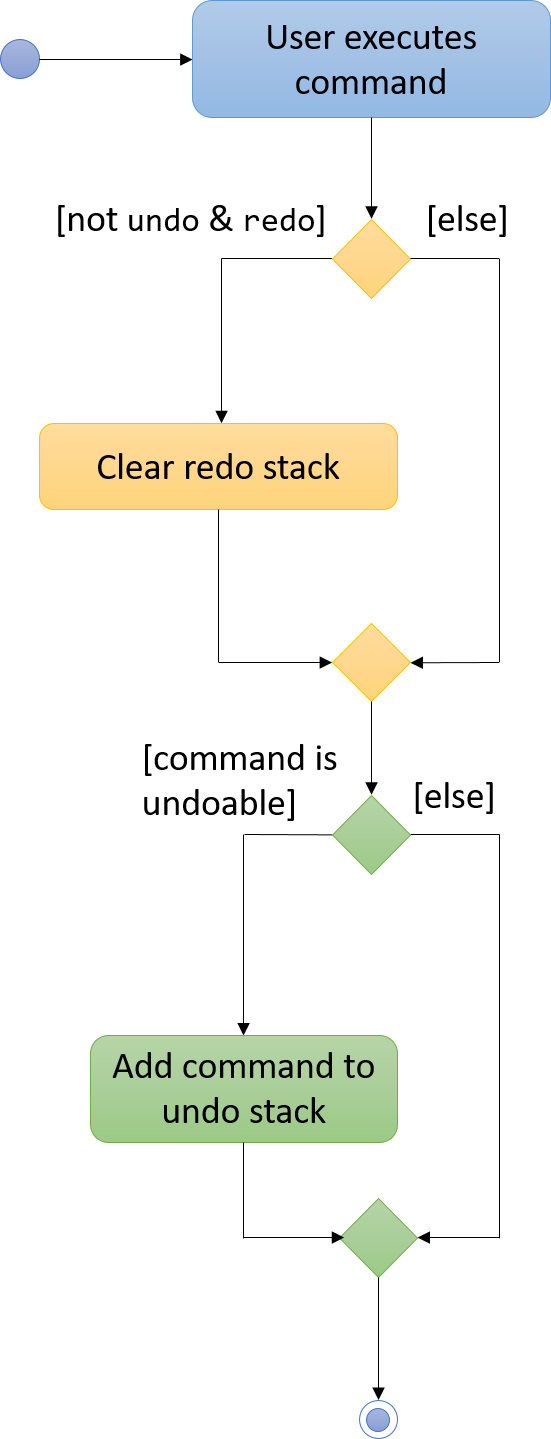
Figure 4.1.7 : The activity diagram describing what happens inside the UndoRedoStack
when the user executes a new
command
4.1.1. Design Considerations
Aspect: Implementation of UndoableCommand
-
Alternative 1 (current choice): Add a new abstract method
executeUndoableCommand()
-
Pros: We will not lose any undone/redone functionality as it is now part of the default behaviour. Classes that deal with
Command
do not have to know thatexecuteUndoableCommand()
exist. -
Cons: Hard for new developers to understand the template pattern.
-
-
Alternative 2: Just override
execute()
-
Pros: Does not involve the template pattern, easier for new developers to understand.
-
Cons: Classes that inherit from
UndoableCommand
must remember to callsuper.execute()
, or lose the ability to undo/redo.
-
Aspect: How undo & redo executes
-
Alternative 1 (current choice): Saves the entire address book.
-
Pros: Easy to implement.
-
Cons: May have performance issues in terms of memory usage.
-
-
Alternative 2: Individual command knows how to undo/redo by itself.
-
Pros: Will use less memory (e.g. for
delete
, just save the parcel being deleted). -
Cons: We must ensure that the implementation of each individual command are correct.
-
-
Aspect: Type of commands that can be undone/redone
-
Alternative 1 (current choice): Only include commands that modifies the address book (
add
,clear
,edit
).-
Pros: We only revert changes that are hard to change back (the view can easily be re-modified as no data are lost).
-
Cons: User might think that undo also applies when the list is modified (undoing filtering for example), only to realize that it does not do that, after executing
undo
.
-
-
Alternative 2: Include all commands.
-
Pros: Might be more intuitive for the user.
-
Cons: User have no way of skipping such commands if he or she just want to reset the state of the address book and not the view.
-
Additional Info: See our discussion here.
-
Aspect: Data structure to support the undo/redo commands
-
Alternative 1 (current choice): Use separate stack for undo and redo
-
Pros: Easy to understand for new Computer Science student undergraduates to understand, who are likely to be the new incoming developers of our project.
-
Cons: Logic is duplicated twice. For example, when a new command is executed, we must remember to update both
HistoryManager
andUndoRedoStack
.
-
-
Alternative 2: Use
HistoryManager
for undo/redo-
Pros: We do not need to maintain a separate stack, and just reuse what is already in the codebase.
-
Cons: Requires dealing with commands that have already been undone: We must remember to skip these commands. Violates Single Responsibility Principle and Separation of Concerns as
HistoryManager
now needs to do two different things.
-
4.2. Logging
We are using java.util.logging
package for logging. The LogsCenter
class is used to manage the logging levels and
logging destinations.
-
The logging level can be controlled using the
logLevel
setting in the configuration file (See Configuration) -
The
Logger
for a class can be obtained usingLogsCenter.getLogger(Class)
which will log messages according to the specified logging level -
Currently log messages are output through:
Console
and to a.log
file.
Logging Levels
-
SEVERE
: Critical problem detected which may possibly cause the termination of the application -
WARNING
: Can continue, but with caution -
INFO
: Information showing the noteworthy actions by the App -
FINE
: Details that is not usually noteworthy but may be useful in debugging e.g. print the actual list instead of just its size
4.3. Configuration
Certain properties of the application can be controlled (e.g App name, logging level) through the configuration file
(default: config.json
).
4.4. Google Maps Search Browser
The google maps search browser enhancement resides within the BrowserPanel
.
It takes in a ReadOnlyParcel
's postal code number substring of the parcel’s address and concatenates it
to the back of Google Map’s search URL prefix to get a URL for the browser to load.
4.4.1. Design Considerations
Aspect: Implementation of Google Maps Search Browser
-
Alternative 1 (current choice): Change browser loadPage URL to Google Map search URL
-
Pros: Its easy to implement new methods to load a new URLs if required to display a different URL.
-
Cons: The map has no other functionality besides searching for the postal code.
-
-
Alternative 2 (future implementation choice): Implementing through Google Maps API
-
Pros: Makes it easier for implementing additional features that utilizes the Maps API which would be required
in future versions of the Ark application. -
Cons: More difficult to implement and integrate into a command line interface.
-
4.5. Tab change mechanism
The tab
command is facilitated by the TabCommand
class within logic
and is an extension of the abstract class
Commands
. TabCommandParser
first checks for valid arguments before returning a new TabCommand
and it executes.
A JumpToTabRequestEvent
event is raised upon execution to be handled by the ParcelListPanel
to display the
switch the selected tab on the UI.
4.5.1. Design Considerations
Aspect: Implementation of tab command
-
Alternative 1: Changing the
select
command to be able to select tabs and parcels-
Pros: Easier for users to understand what the command does by its name alone since
select tab 2
orselect index 2
is descriptive of the commands actions+ -
Cons: Harder to implement since it would require a large change of
SelectCommand
class and its related files to take in a third argument, and user would have to type additional word every select
-
-
Alternative 2 (current choice): Creating a new command called
tab
-
Pros: Easier to implement and less words for the user to input
-
Cons: Just the word "tab" alone is not very descriptive of the commands function.
-
4.6. Overdue parcels popup window
The overdue parcels popup window is facilitated by the PopupOverdueParcelsWindow
which extends UiPart<Region>
within ui
. It creates a new dialogStage
which is set to always show
on top of MainWindow
.
A new PopupOverdueParcelsWindow
is created and set to show in UiManager
on start
and takes in an
ObservableList<ReadOnlyParcel>
from logic
as an argument. This popup window only shows if the method
hasOverdueParcels
in UiManager
returns true to signify the presence of parcels with OVERDUE
status in the
uncompleted parcels list. Another method getNumOverdueParcels
is then used to get the integer number of
overdue parcels to display in the popup window.
Javafx
's animation API for PauseTransition
is then used to hide
the window after seven seconds.
4.6.1. Design Considerations
Aspect: When the popup window shows
-
Alternative 1 (current choice): Only at every startup of the Ark application
-
Pros: Only needs to check the condition to show the popup once and is easy to implement a single check in
UiManager
. -
Cons: Only notifies users once an on startup of Ark only so users would not get any more notifications if they keep their application open constantly
-
-
Alternative 2: Check uncompleted parcel list after every command and show popup if overdue parcels are found
-
Pros: Users will be immediately notified of overdue parcels whenever parcel list is changed
-
Cons: May not be necessary since the user themselves would be the ones to
add
oredit
the parcel causing theOVERDUE
status, hence they would already know of the existence of these overdue parcels.
-
4.7. Backup Mechanism
The back up mechanism is facilitated by a backup(addressBook:AddressBookStorage)
method within the StorageManager
class. It supports the backup of data in Ark.
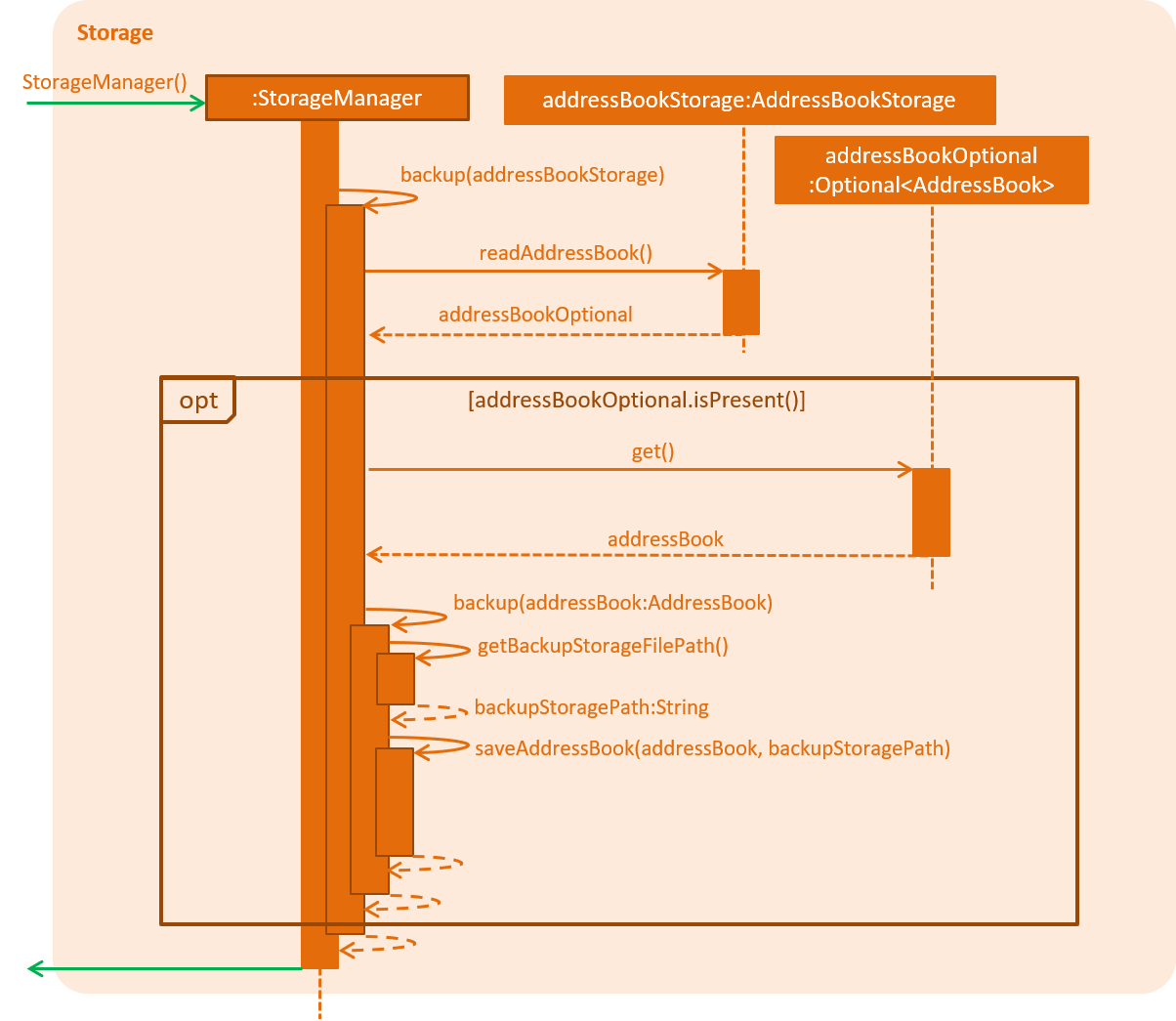
Diagram 4.7.1 : Sequence diagram describing the operation of the storageManager
when it is initialized
As seen in the sequence diagram above, the backup(addressBook:AddressBookStorage)
method is called when
storageManager
is initialised in MainApp#init()
. The MainApp#init()
is called when Ark launches.
From the diagram above, the backup()
method is called when storageManager
is initialized. This backup method first
checks if there is a valid save file loaded into Ark. If there is a save file loaded into Ark,
the backup(addressBook:AddressBook)
method will be called. Otherwise, Ark does not backup the missing save file.
To save the backup data, Ark first retrieves the backup file path by calling the getBackupStorageFilePath()
method.
Then, it will call the saveAddressBook(addressBook, backupStoragePath)
method to save a backup of Ark at the retrived
backup file path.
4.7.1. Design Considerations
Aspect: Implementation of StorageManager#backup()
-
Alternative 1 (current choice): use the
saveAddressBook()
method to implement logic.-
Pros: It becomes easier to implement method rather than writing out a separate logic for
backup()
. It makes updates easier since enhancements to saving Ark insaveAddressBook()
will also enhancebackup()
. -
Cons: This implementation increases the coupling of
backup()
andsaveAddressBook()
where changes insaveAddressBook()
are likely to cause changes inbackup()
.
-
-
Alternative 2: Separate the implementation of
backup()
fromsaveAddressBook()
-
Pros: Reduced coupling of
saveAddressBook()
andbackup()
and allows the backup file to be saved at a different location from the main save file. This prevents the backup file from being corrupted if the folder of the main save file becomes corrupted. -
Cons: More tedious to implement and maintain
backup()
since enhancements to the saving feature has to be implemented in bothsaveAddressBook()
andbackup()
-
Aspect: Trigger to execute the backup mechanism
-
Alternative 1 (current choice): Automatically backup data on launch.
-
Pros: This implementation ensures that the if the user corrupts the data of Ark during a session. The user will be able to revert to the start of the session, which is likely to be a functional copy of his original save file.
-
Cons: This does not give the most recent copy of the data of the Ark if many changes were made in a single session.
1* Alternative 2: Backup data every few minutes -
Pros: Provides a very recent copy of the data on Ark.
-
Cons: More tedious and difficult to implement. User may also be running another process at that point of time. This could cause a bottleneck if there is a lot of data to be saved, and multiple backup calls will be queued one after the other if the previous backup process is called even before the current one has finished running.
-
-
Alternative 3: Backup data after a fixed number of
UndoableCommand
.-
Pros: Provides a very recent copy of the data on Ark.
-
Cons: More tedious and difficult to implement. Difficult to determine the optimal amount of data to restore. If the corruption of the data is caused by a series of commands, it becomes difficult to provide reasonable assurance that the backup file provides a functional copy of the data of Ark.
-
Aspect: Case: Backup if main storage file is not present
-
Alternative 1 (current choice): Ark does not backup if it cannot read the main storage file
-
Pros: Backup data will not be overwritten in the event that Ark is not able to read the designated save file for reasons such as the save file being corrupted/missing.
-
Cons: Additional overhead to check if Ark is able to read the save file.
-
-
Alternative 2:Back up even if the main storage file does not exist or cannot be read by Ark.
-
Pros: Less overhead needed to check if Ark is able to read the save file.
-
Cons: Backup data could be overwritten in the event the designated save file is corrupted/missing.
-
4.8. Import Mechanism
To use this command, you can type import
and the name of your file into the CommandBox
. e.g. import ark_save
The import
mechanism allows users to import parcels from valid storage files stored in a .xml
format. This mechanism
allows users to add multiple parcels stored in the imported storage file into the running instance of Ark. This
mechanism is facilitated by the readAddressBook()
method within AddressBookStorage()
to
load the parcels stored in the storage file and the ModelManager#addAllParcels
method to add the parcels in
the storage file into the running instance of Ark
.
Since the import
mechanism modifies the data stored in Ark
, it should be an extension of the UndoCommand
. Thus,
it inherits from the UndoableCommand
interface rather than inheriting direclty from the Command
interface.
The following sequence diagram describes the sequence of events that occur when you enter 'import ark_save' into the
CommandBox
:
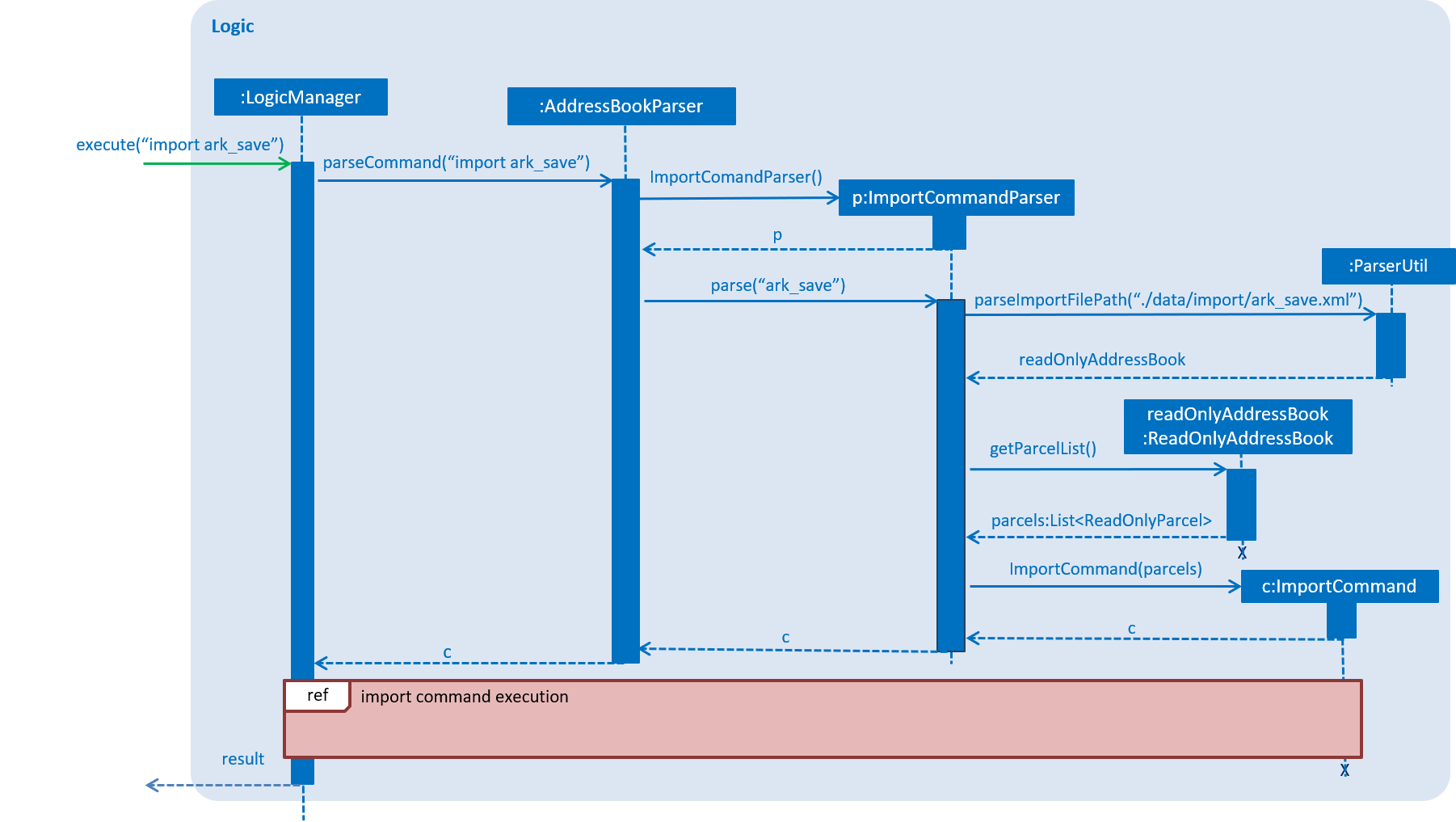
Figure 4.8.1 : Sequence diagram describing the operation of import
when it is executed
As seen in the sequence diagram above, the command is first parsed to create an ImportCommandParser
. This parser
takes the arguments of the import command ("ark_save")
as the name of the file to import and converts it to a
full file path string ("./data/import/ark_save.xml")
to locate the file to import. Thereafter, it loads the file
to import into Ark and reads the data. This returns a list of parcels that are used in create an ImportCommand
.
When the command is executed, the following sequence of events take place:
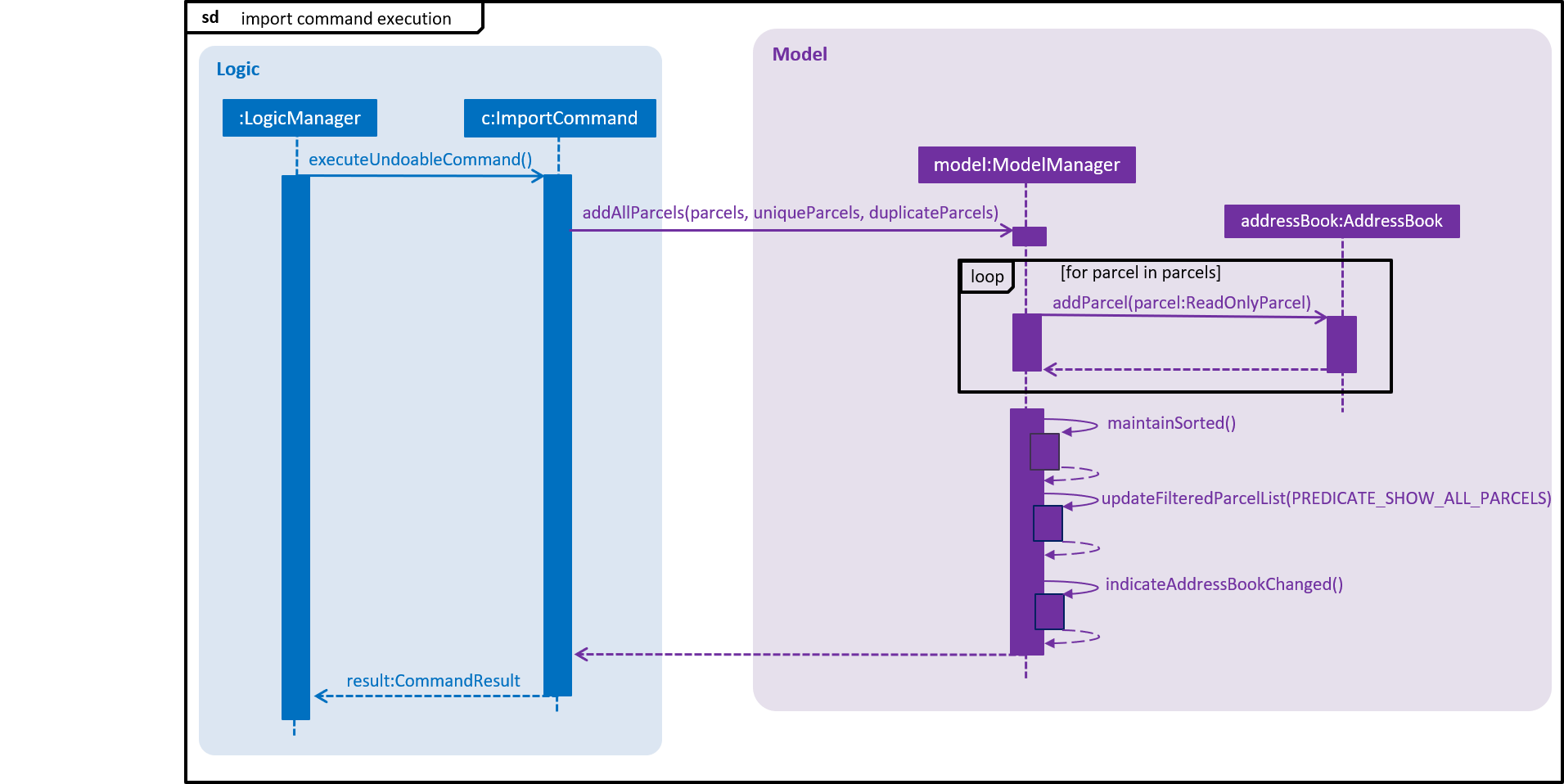
Figure 4.8.2 : Reference frame for the execution of the Import Command
As seen in the diagram above, when the command is executed, the executeUndoableMethod
methods calls
ModelManager#addAllParcels
method. In this method, all unique parcels are added into the running instance of Ark.
If the parcel is not unique such that it will create a duplicate parcel in the current instance of Ark,
the parcel is ignored and the process continues until the last parcel has been added.
The file to be imported has to be stored in the ./data/import folder. i.e. calling import ark_save will import the
file ./data/import/ark_save.xml .If the user enters a file name that contains characters other than alphanumeric characters or imports a file that is not in a .xml format, the command will throw an Exception.
|
The ImportCommand will only add non-duplicate parcels. Duplicate parcels are ignored.If all the parcels to be imported into Ark are duplicates, then no parcels are imported and an Exception is thrown. If the file to import is missing or empty, a CommandException will be thrown
|
4.8.1. Design Considerations
Aspect: Implementation of ImportCommand
-
Alternative 1 (current choice): using
readAddressBook()
to implement the logicImportCommand
**-
Pros: It becomes easier to implement method rather than writing out a separate logic to import files. It makes updates easier since enhancements to
readAddressBook()
will also enhance the import command such as more supported save file formats. -
Cons: This implementation increases the coupling of the
readAddressBook()
andImportCommand
such that changes inreadAddressBook()
is likely to cause a change inImportCommand
.
-
-
Alternative 2: Implement a parsing logic for
ImportCommand
.-
Pros: Reduced coupling of
readAddressBook()
andImportCommand
. This gives the developers more freedom on adding more file formats that can be imported. -
Cons: More tedious to implement and maintain
ImportCommand
since enhancements to thereadAddressBook()
feature has to be manually implemented inImportCommand
as well.
-
Aspect: Arguments to import files
-
Alternative 1 (current choice): Imports save files from only one location
-
Pros: User will only store his save files at one location, he will not store them at random locations and lose track of them. User only has to type the name of the file and does not need to type the full file path to locate the file. i.e. the user does not need to type
./data/import/Ark.xml
. -
Cons: The user has restrictions on where he can import files from.
-
-
Alternative 2: User can load the files from any directory
-
Pros: Allows user to import from his own archived folders anywhere in this computer.
-
Cons: More tedious for the user to type in the full file path to locate the .xml file that he wants to import.
-
Aspect: Allowed file names that can be imported
-
Alternative 1 (current choice): File Names can only contain alphanumeric and underscore characters and be in the
.xml
format.-
Pros: Users need to constraint their file naming to contain more semantic names rather than having non-alphanumeric or non-underscore characters in their naming of Ark save files.
-
Cons: The user has restrictions on the file naming conventions he can use to name the save files that he wants to import.
-
-
Alternative 2: No file name check
-
Pros: Allows user to name his files following any conventions and be successfully imported into Ark.
-
Cons: Makes Ark vulnerable to simple directory traversals where user can access files outside the
data/import/
directory.
-
4.9. Tab autocomplete mechanism
The tab autocomplete mechanism is facilitated by the autocompleter
package. The structure of the autocompleter
package can be seen in the class diagram below:
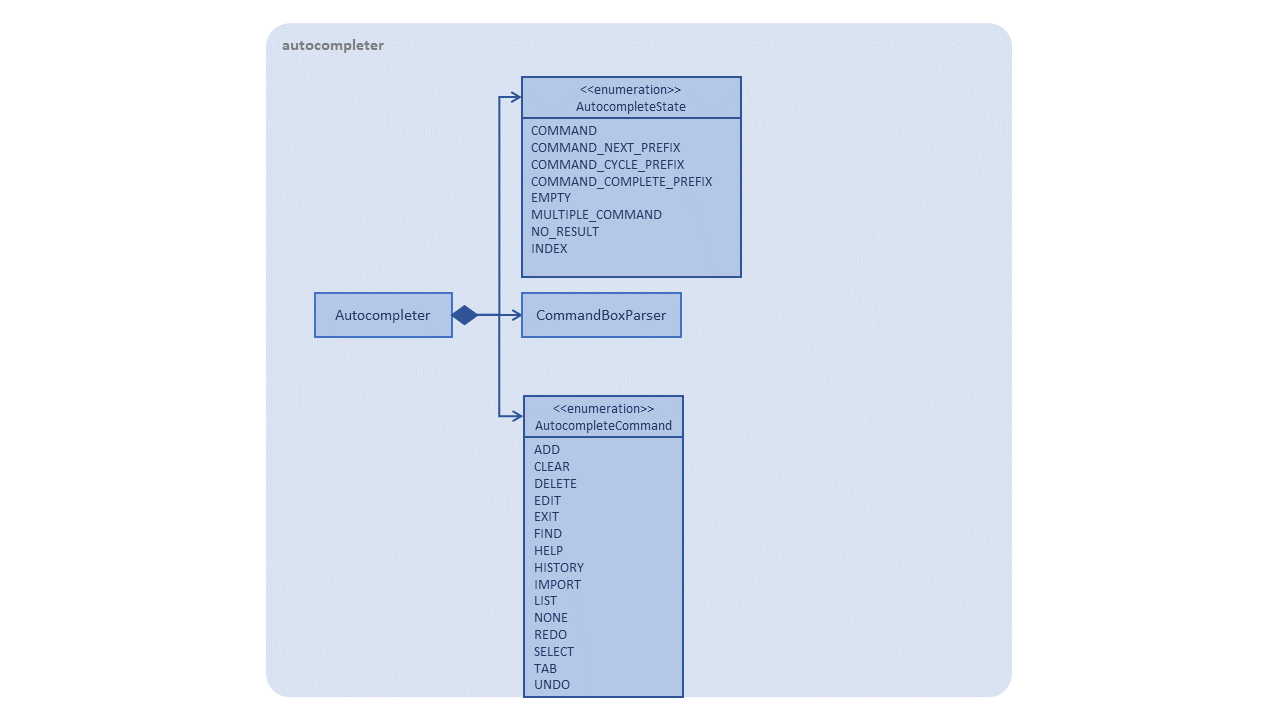
Figure 4.9.1 : Class diagram of the autocomplete package
The role of the CommandBoxParser
Class is to parse the text in the CommandBox
to extract commands and arguments as well
as to find missing prefixes.
The role of the AutocompleteCommand
Enum is to keep track of the current command that the Autocompleter
recognizes.
The rote of the AutocompleteState
Enum is to keep track of the current state of the Autocompleter
.
The Autocompleter
class is the main entry point into the package. An instance of the Autocompleter
class is
instantiated inside the CommandBox
class on start up. Inside the CommandBox
, an event listener is
attached to the TextField
which calls the updateAutocompleter
method whenever the text inside it is
changed. The updateAutocompleter
method then calls the updateAutocompleter
method in the Autocompleter
which
updates the state of the Autocompleter
according to the diagram below:
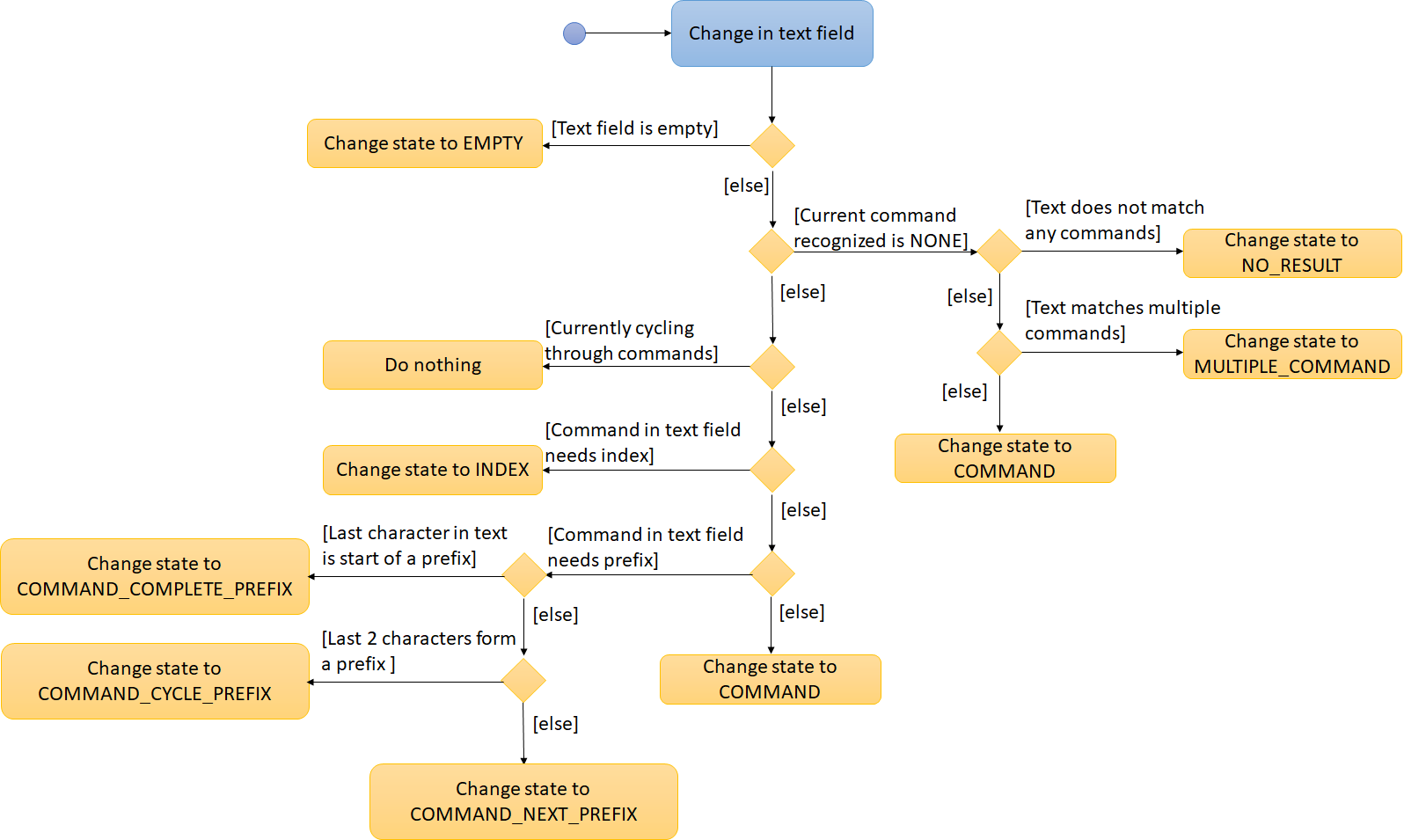
Figure 4.9.2 : Activity diagram of the updateAutocompleter
method
Besides updating the state, the updateState method also updates the possibleAutocompleteOptions
list. In the case
where there are multiple commands available, this will contain all the possible options. In the case where multiple
prefixes are available, this will contain the missing prefixes. These options are accessed using the resultIndex
which
is either incremented with wrap-around or reset depending on the state of the Autocompleter
when autocomplete
is called.
The countingIndex is used to keep track of the index field for commands that need it. Its maximum size is the
size of the current ActiveList in the model. It is either incremented with wrap-around or reset depending on
the state of the Autocompleter
when autocomplete
is called.
When tab is entered by the user, the autocomplete
method is called through the processAutocompelete
in the
Command box which then updates the text field according to the diagram below.
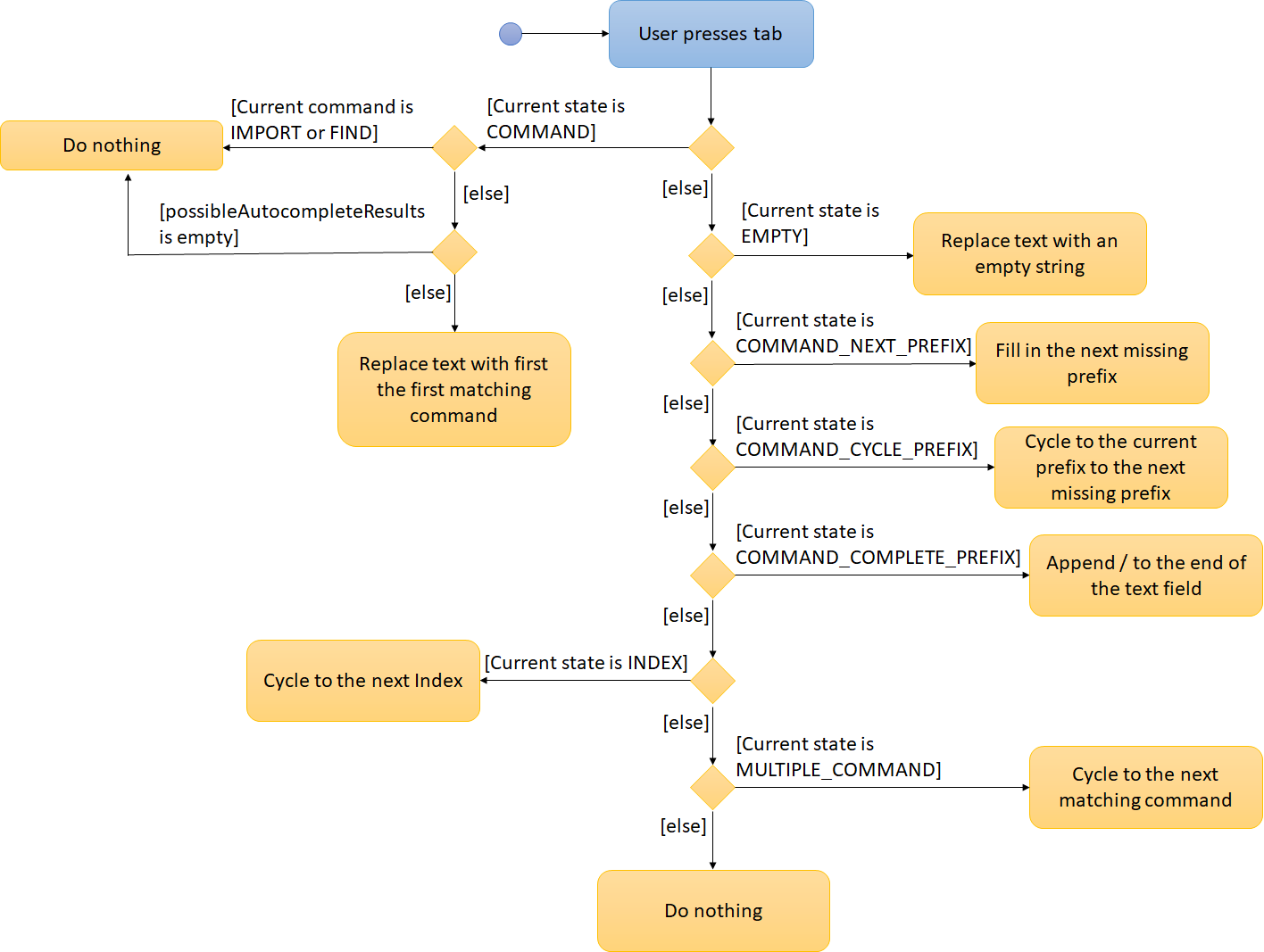
Figure 4.9.3 : Activity diagram of the autocomplete
method
4.9.1. Design Considerations
Aspect: autocomplete interface
-
Alternative 1 (current choice): A unix like tab auto-completion mechanism that allows users to cycle through options.
-
Pros: More intuitive and non-intrusive interface, which improves user experience.
-
Cons: Harder to implement, the whole autocompleter would be built from the ground up.
-
-
Alternative 2: A drop down box that gives users suggestions on the options they have.
-
Pros: Can be easily implemented by using a ComboBox in JavaFX.
-
Cons: Highly intrusive and isn’t as intuitive as a tab autocomplete. The ComboBox response time might also be too slow for people who type fast.
-
Aspect: Implementation of autocomplete
-
Alternative 1 (current choice): Create a new
Autocompleter
class to implementautocomplete
and its helper functions.-
Pros: Single Responsibility Principle (SRP) is maintained
-
Cons: More tedious to implement and test since the feature is implemented in both
Autocompleter
andCommandBox
. Also creates coupling between theAutocompleter
andCommandBox
.
-
-
Alternative 2: Implement
autocomplete
insideCommandBox
-
Pros: Easier to test since
CommandBoxTest
has already been set up and implemented. -
Cons:
CommandBox
class now has multiple responsibilities, which violates SRP.
-
4.10. Tracking Number Field
Parcels have tracking numbers for delivery vendors to keep track of the parcels that they send out on a daily basis. This feature is important because a single person can have many parcels belonging to him. Tracking numbers are used to differentiate between the different parcels that are going to be delivered to the same person. Tracking numbers also serve as a better way of narrowing down and pinpointing parcels of interest since these numbers are likely to be more unique than names in a localized region.
Presently, the Tracking Number Field only has support for Registered Article tracking numbers belonging to SingPost.
You can read more about their Registered Article tracking number
here.
|
4.10.1. Design Considerations
Tracking Numbers implementation.
-
Alternative 1 (current choice): Ark allows duplicate
TrackingNumber
entries.-
Pros: In the event that the number of parcels in the users' inventory exceeds the number of possible
TrackingNumber
entries, the user will still be able to add the parcel into Ark. There has been instances where tracking numbers were reused. Moreover, different delivery companies have different policies on how the tracking numbers` are used. Therefore, it is difficult to implement a general policy forTrackingNumber
entries. -
Cons: Less intuitive to users.
-
-
Alternative 2: Ark only allows non-duplicate
TrackingNumber
entries.-
Pros: More intuitive for users.
-
Cons: Impossible to add parcel with duplicate entry even if the parcel does have that specific
Tracking Number
. This issue can occur during festive periods such as Christmas when the number of parcels can exceed the number ofTrackingNumber
entries.
-
4.11. Postal Code Field
The postal code field is represented using the PostalCode
class. The PostalCode
field is implemented as part of the
Address
class. The PostalCode
class stores the postal address the address text. It only accepts values of
s
or S
followed by 6 digits. The PostalCode
will generate a String
to query Google Maps when the select
command is executed or a when a parcel is selected.
Presently, the PostalCode field still does a very relaxed validation and does not completely ensure that the postal
code exists even though it might meet the criteria above. The team is working on producing a database of postal codes
in Singapore by querying the Google Maps Distance Matrix API. In the meantime, it is assumed that users will enter
the correct postal code.
|
4.11.1. Design Considerations
Implementation of Postal Code
-
Alternative 1 (current choice):
Postal Code
is designed as a part of theAddress
class.-
Pros: This composition relationship is more intuitive. If an
Address
is deleted, its correspondingPostalCode
is also deleted. -
Cons: -
-
-
Alternative 2: Separate the
PostalCode
class from theAddress
class-
Pros: -
-
Cons: When an
Address
is deleted, its correspondingPostalCode
has to be searched and deleted as well. This results in more overhead.
-
Valid inputs to the PostalCode
class
-
Alternative 1 (current choice): `Postal code accepts postal district codes above 80. (first two digits of postal code)
-
Pros: This ensures that when new postal code districts are added into Singapore, the user will be able to add the postal codes from the new postal code district without receiving an error.
-
Cons: The user might enter postal codes that belong to a non-existent district e.g. S810000
-
-
Alternative 2: Reject postal codes with postal district codes above 80.
-
Pros: The user will have a stricter validation of his postal code.
-
Cons: If a new postal district is added, e.g. 81, and there is parcel that has a postal code belonging to the district, Ark will reject that parcel from being entered,
-
4.12. Status field
Status
represents the current stage of delivery that a parcel is at. As seen in the class diagram below,
Status
implements an Enumeration
interface and it has the four possible values:
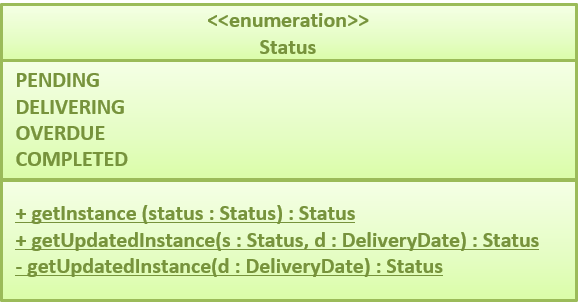
Figure 4.12.1: Status Class Diagram
The following are the descriptions for the four possible values of Status
:
Status | Description |
---|---|
PENDING |
This means that the parcel has not been delivered and has not passed the date it is supposed to be delivered by. |
DELIVERING |
This means that the parcel is currently working being delivered to its destination address. |
COMPLETED |
This indicates that the parcel has been successfully delivered to its destination. |
OVERDUE |
This state indicates that the parcel has not been delivered and has passed its due date. |
As seen in the class diagram, the getInstance()
method retrieves the static instance of Status
based on the status
String input. For example, getInstance("pending")
will return the PENDING Status
. Additionally, Status is updated
automatically when a parcel is edited or added. The Status
of parcels in Ark will also be updated when Ark first launches.
This is done through the getUpdatedInstance(s : Status, d : DeliveryDate)
method and the overloaded method
getUpdated(d : DeliveryDate)
method.
The automatic Status
update updates based on the comparison of today’s date to the DeliveryDate
object given as the
parameter of getUpdatedStatus()
. If today’s date is after the date indicated in the DeliveryDate
object, the method
will return an OVERDUE Status
. Otherwise, it will return a PENDING Status
. The Status
update only works for
Status
values of PENDING
and OVERDUE
. COMPLETED
and DELIVERING Status
are not updated.
4.12.1. Design Considerations
Implementation of Status
-
Alternative 1 (current choice): Status implements an Enumeration interface.
-
Pros:
Status
has a fixed number of values. The user should also not be allowed to create newStatus
objects. This also standardizes the naming conventions used to describe the same delivery status in Ark. -
Cons: Less options for the user to alter the
Status
values
-
-
Alternative 2: Allow the user to define any
Status
they wish.-
Pros: Users have more versatility on naming conventions
-
Cons: It becomes more difficult to import data files since different users may use different terminologies to describe the same
Status
of the parcel.
-
Updating of Status
-
Alternative 1 (current choice): Separate
Status
update from retrieving an instance of Status-
Pros: By separating the update and retrieval, we are using the Separation of Concerns Principle,
getInstance()
checks if the String input is a validStatus
and returns it. On the other hand,getUpdatedInstance()
receives a validStatus
and returns an updatedStatus
with respect to theDeliveryDate
provided as a parameter. Easier to test these methods and integrate the automaticStatus
update into the codebase. -
Cons: More overhead.
-
-
Alternative 2: Update
Status
ingetInstance()
.-
Pros: Less overhead and more intuitive.
-
Cons: More tedious to code because a
DeliveryDate
object has to be instantiated before user is able to retrieve an updatedStatus
.
-
4.13. Delivery Date field
Delivery Date
is used to indicate the delivery date that the parcel must be delivered by.
The dates are only accepted if they are in the valid format DD-MM-YYYY or understandable by Ark.
Ark is able to recognise various forms of dates as shown in the table below but the dates in the Ark are formatted as DD-MM-YYYY. However, invalid inputs such as a phone number or symbols still will be rejected.
Current date as of writing is 12 November 2017.
User input | Date parsed by Ark |
---|---|
01-01-2017 |
01-01-2017 |
01/01/2017 |
01-01-2017 |
01.01.2017 |
01-01-2017 |
01-01-17 |
01-01-2017 |
First day of 2017 |
01-01-2017 |
The day before yesterday |
10-11-2017 |
Yesterday |
11-11-2017 |
Today |
12-11-2017 |
Tomorrow |
13-11-2017 |
The day after tomorrow |
14-11-2017 |
Three days from now |
15-11-2017 |
Four days later |
16-11-2017 |
Seventeenth of November |
17-11-2017 |
This Friday |
17-11-2017 |
Next Friday |
24-11-2017 |
Christmas Eve |
24-12-2017 |
A week before Christmas Eve |
17-12-2017 |
A year from now |
12-11-2018 |
Friday of the second week of January |
Query too complicated, date defaults to today |
123456789 |
Invalid date error shown |
!@#$%^&*() |
Invalid date error shown |
The parcel list is maintained in sorted order by comparing their delivery dates, with the earliest on top.
The following sequence diagram shows how the delivery date is parsed to PrettyTime's parser:
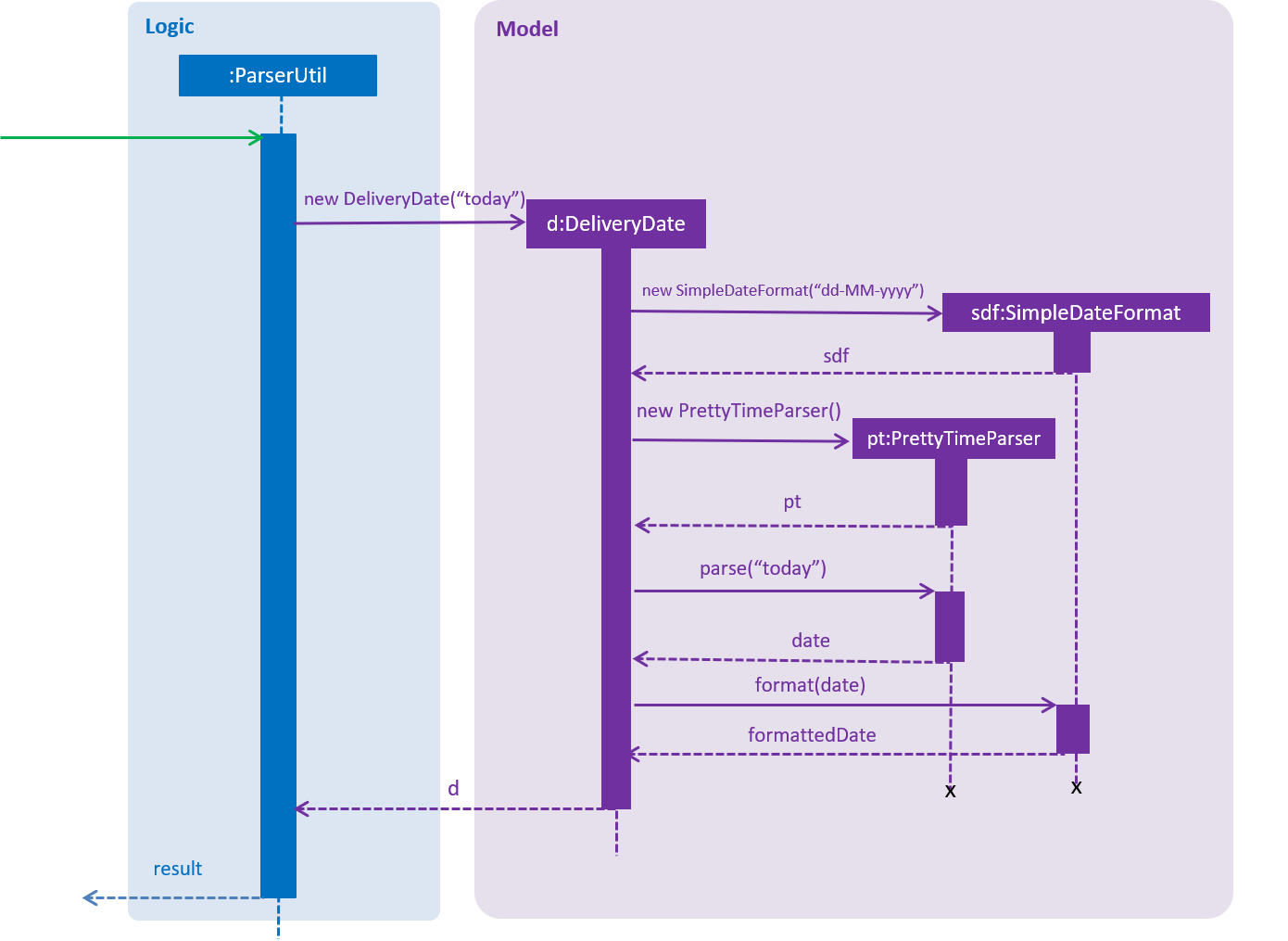
Figure 4.13.1 : Sequence diagram describing the parsing of delivery date when edit 1 d/today
is executed
As seen in the sequence diagram above, a request comes in to ParserUtil to parse the delivery date. A new
DeliveryDate is then created and within it we instantiate a SimpleDateFormat object with the desired date
format and an instance of PrettyTimeParser. We then request for PrettyTimeParser to parse the string today
and subsequently pass it’s result into our SimpleDateFormat to format our date in the way we’ve defined, dd-MM-yyyy
.
After the date has been formatted, we store it within the DeliveryDate object to be returned to ParserUtil and
subsequently returned to whichever method which called it.
4.14. Tags Field
Tags
are used to indicate how the parcel should be handled. The Tags
field can contain one or more of the following Tag
:
Tag | Description |
---|---|
FROZEN |
This means the parcel should be refrigerated as its contents are temperature sensitive. |
FLAMMABLE |
This means that the parcels' contents are highly flammable and should be kept away from heat. |
HEAVY |
This indicates that the parcel is heavy and may require additional manpower to deliver. |
FRAGILE |
This state indicates that the parcels' contents can be broken easily and requires additional care when handling. |
4.14.1. Design Considerations
Implementation of Tag
-
Alternative 1 (current choice): Tag implements the Enumeration interface.
-
Pros:
Tag`s has fixed values. The user should also not be allowed to create new `Tag
objects. -
Cons: Less options for the user to alter the
Tag
values
-
-
Alternative 2: Allow the user to define any `Tag`s they wish.
-
Pros: Users have more versatility on naming conventions
-
Cons: It becomes difficult for delivery personnel to keep track of the tags since different personnel might use different tag names to refer to the same tag.
-
4.15. Maintain sorted mechanism
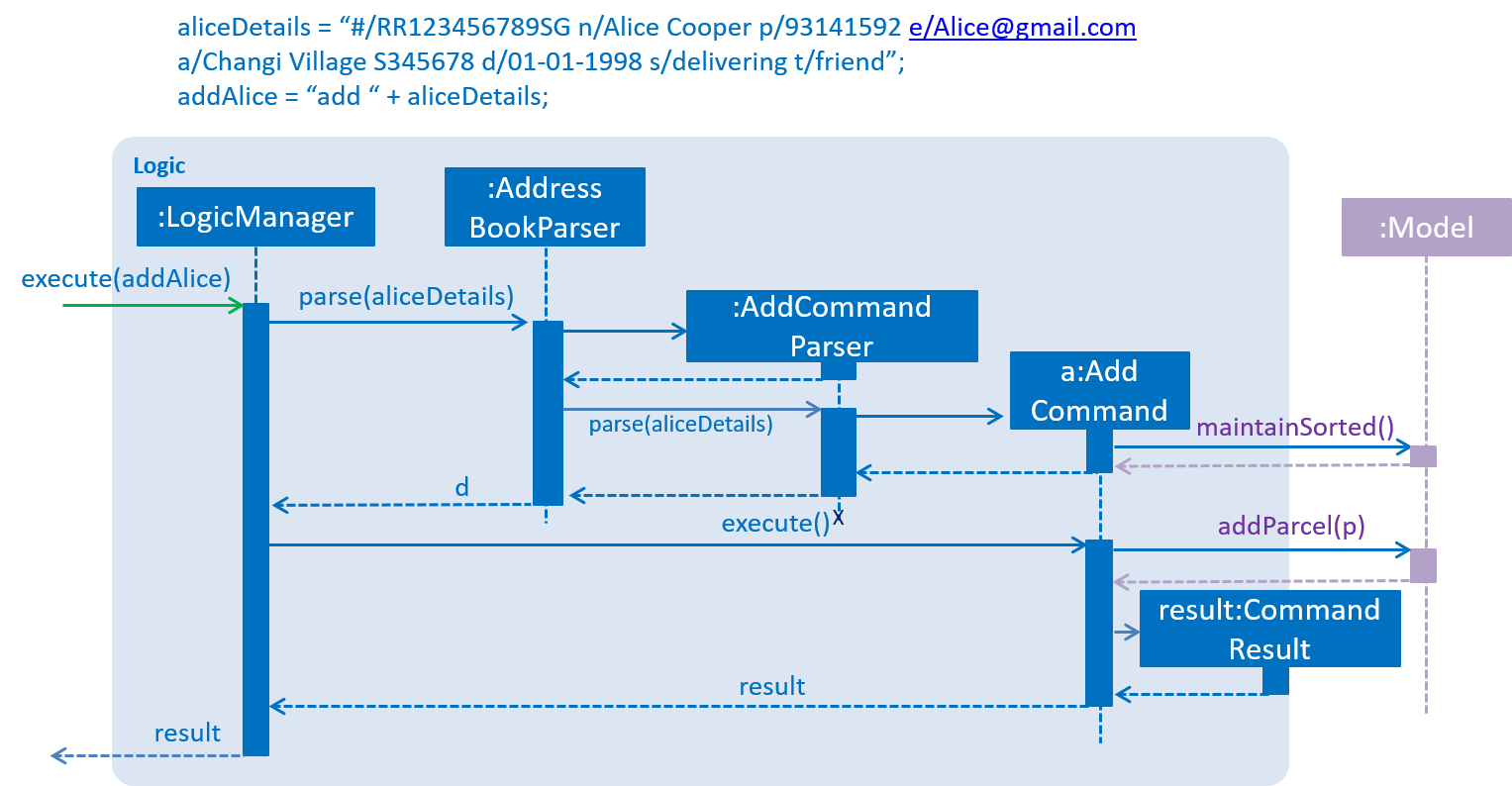
Figure 4.15.1 : Adding Alice to Ark, maintainSorted is actually called and returns void.
The list of parcels in Ark is maintained to be always in sorted order according to delivery dates,
with the earliest being on the top. This is so that the user will be able to look at the more
pertinent deliveries.
The list is sorted whenever a parcel is added or edited. This is
because these commands are the ones that might possibly cause the new parcel to be placed
in the wrong position.
Ark has two tabs, one for uncompleted parcels, the other for completed parcels. Whenever add
and edit
commands
are executed, the newly added or edited parcel gets selected. If the status of the newly added or edited parcel is
not on the current active list, a tab switch needs to happen. This is taken care off by the selection mechanism within
the Model Manager class.
4.15.1. Design Considerations
Aspect: Implementation of maintainSorted
-
Alternative 1 (current choice): Constant sort the list of parcels whenever there is a change that potentially could disrupt the order of the list.
-
Pros: Intuitive and guarantees that list is sorted in the right order
-
Cons: Many commands have to be changed
-
-
Alternative 2: Insert the new / edited parcel to fit into the sorted list.
-
Pros: Use less computation as the list of parcels is already sorted.
-
Cons: More difficult to implement as we’ll need to implement our own sorting algorithm as opposed to just using the built in sorting methods.
-
4.16. ArkBot: Telegram Bot Interface for Ark
ArkBot is written using TelegramBots, a Java library written by rubenlagus to create bots using Telegram Bots API.
At present the following commands have an equivalent in ArkBot: add
delete
list
find
undo
redo
help
. ArkBot also has the added functionality of complete
which is merely a wrapper around the edit
command to mark parcel
deliveries as complete. This is especially useful for our delivery man.
Like all Telegram Bots, each command must be prefixed with a /
character. So if I were to want to trigger the help
command, I would send /help
to ArkBot.
At present, all messages and commands to ArkBot are sent directly to Ark where they are processed and the commands and arguments are formatted as Ark commands and subsequently executed.
If we enter the complete
command into ArkBot without any parameters, we enter listen
mode. In listen
mode, ArkBot is waiting for a QR to be sent to be analysed. The image is then downloaded and using the
zxing barcode scanning library for Java, the information is unwrapped and parsed into
the edit command to change the status of the parcel to COMPLETED
.
4.16.1. Design Considerations
Aspect: Implementation of ArkBot
-
Alternative 1 (current choice): Parse the arguments from the user into various commands as needed. If there any errors that surface, reply user with default error message.
-
Pros: Functions of commands on Ark remain the same, reusing current implementation
-
Cons: Error catching is difficult, it is difficult to test and slower as information is handle by many parties.
-
-
Alternative 2: Program each command from the Bot to directly interface with Model and Logic Managers on Ark
-
Pros: Quicker and more director way of communication. Less reliant on Ark commands.
-
Cons: More difficult to implement as we’ll need to implement our own version of all the command on Ark.
-
5. Documentation
We use asciidoc for writing documentation.
We chose asciidoc over Markdown because asciidoc, although a bit more complex than Markdown, provides more flexibility in formatting. |
5.1. Editing Documentation
See UsingGradle.adoc to learn how to render .adoc
files locally to preview
the end result of your edits. Alternatively, you can download the AsciiDoc plugin for IntelliJ, which allows you to
preview the changes you have made to your .adoc
files in real-time.
5.2. Publishing Documentation
See UsingTravis.adoc to learn how to deploy GitHub Pages using Travis.
5.3. Converting Documentation to PDF format
We use Google Chrome for converting documentation to PDF format, as Chrome’s PDF engine preserves hyperlinks used in webpages.
Here are the steps to convert the project documentation files to PDF format.
-
Follow the instructions in UsingGradle.adoc to convert the AsciiDoc files in the
docs/
directory to HTML format. -
Go to your generated HTML files in the
build/docs
folder, right click on them and selectOpen with
→Google Chrome
. -
Within Chrome, click on the
Print
option in Chrome’s menu. -
Set the destination to
Save as PDF
, then clickSave
to save a copy of the file in PDF format. For best results, use the settings indicated in the screenshot below.
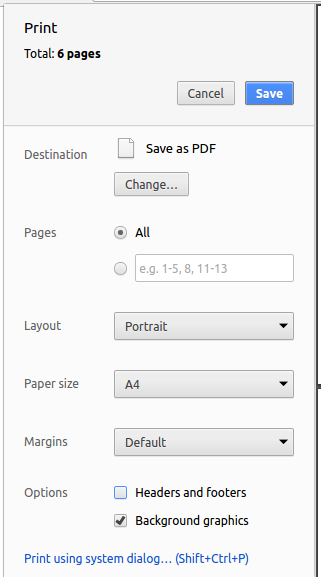
Figure 5.3.1 : Saving documentation as PDF files in Chrome
6. Testing
6.1. Running Tests
There are three ways to run tests.
The most reliable way to run tests is the 3rd one. The first two methods might fail some GUI tests due to platform/resolution-specific idiosyncrasies. |
Method 1: Using IntelliJ JUnit test runner
-
To run all tests, right-click on the
src/test/java
folder and chooseRun 'All Tests'
-
To run a subset of tests, you can right-click on a test package, test class, or a test and choose
Run 'ABC'
Method 2: Using Gradle
-
Open a console and run the command
gradlew clean allTests
(Mac/Linux:./gradlew clean allTests
)
See UsingGradle.adoc for more info on how to run tests using Gradle. |
Method 3: Using Gradle (headless)
Thanks to the TestFX library we use, our GUI tests can be run in the headless mode. In the headless mode, GUI tests do not show up on the screen. That means the developer can do other things on the Computer while the tests are running.
To run tests in headless mode, open a console and run the command gradlew clean headless allTests
(Mac/Linux:
./gradlew clean headless allTests
)
6.2. Types of tests
We have two types of tests:
-
GUI Tests - These are tests involving the GUI. They include,
-
System Tests that test the entire App by simulating user actions on the GUI. These are in the
systemtests
package. -
Unit tests that test the individual components. These are in
seedu.address.ui
package.
-
-
Non-GUI Tests - These are tests not involving the GUI. They include,
-
Unit tests targeting the lowest level methods/classes.
e.g.seedu.address.commons.StringUtilTest
-
Integration tests that are checking the integration of multiple code units (those code units are assumed to be working).
e.g.seedu.address.storage.StorageManagerTest
-
Hybrids of unit and integration tests. These test are checking multiple code units as well as how the are connected together.
e.g.seedu.address.logic.LogicManagerTest
-
6.3. Troubleshooting Testing
Problem: HelpWindowTest
fails with a NullPointerException
.
-
Reason: One of its dependencies,
UserGuide.html
insrc/main/resources/docs
is missing. -
Solution: Execute Gradle task
processResources
.
7. Dev Ops
7.1. Build Automation
See UsingGradle.adoc to learn how to use Gradle for build automation.
7.2. Continuous Integration
We use Travis CI and AppVeyor to perform Continuous Integration on our projects. See UsingTravis.adoc and UsingAppVeyor.adoc for more details.
7.3. Making a Release
Here are the steps to create a new release.
-
Update the version number in
MainApp.java
. -
Generate a JAR file using Gradle.
-
Tag the repo with the version number. e.g.
v0.1
-
Create a new release using GitHub and upload the JAR file you created.
7.4. Managing Dependencies
A project often depends on third-party libraries. For example, Address Book depends on the
Jackson library for XML parsing. Managing these dependencies can be automated
using Gradle. For example, Gradle can download the dependencies automatically, which is better than these alternatives.
a. Include those libraries in the repo (this bloats the repo size)
b. Require developers to download those libraries manually (this creates extra work for developers)
8. Known Bugs
8.1. Header scaling bug
Currently, the headers for the Parcel list do not scale with it when the window size is changed, a fix for it is in the works and is expected to be out in v1.6.
8.2. Redo command bug
If you find (eg find Roy), then do a command (eg delete 1), then go back to the list, then undo and redo, the wrong person will be deleted. This bug is from the original address book. A fix for it is in the works and is expected to be out in v1.6
Appendix A: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
|
new user |
see usage instructions |
refer to instructions when I forget how to use the App |
|
ongoing user |
have a backup of my addressbook data |
restore my addressbook if the storage file becomes corrupted |
|
user |
add a new parcel |
|
|
user |
delete a parcel |
|
|
user |
find a parcel by name |
locate details of parcels without having to go through the entire list |
|
user |
the browser to automatically search for the address of the selected parcel in Google Maps |
so that I can automatically get information on how to get to an address automatically, on click. |
|
delivery company |
be able to keep track of my deliveries |
deliver the packages on time |
|
delivery company |
be alerted for any deliveries to be done today |
deliver the packages on time |
|
delivery company |
sort my deliveries by date |
know which packages are more urgent to handle |
|
delivery company |
add a list of deliveries in one shot using Comma Separated Values |
conveniently parse information from other sources |
|
delivery company |
check for deliveries close to deadline |
better prepare for busy periods |
|
delivery company |
archive completed deliveries |
refer to them in the future |
|
new user |
to have an autocomplete for the commands |
I do not need to remember the format of commands |
|
lazy user |
to be notified of the most optimal path of completing my deliveries based on travelling distance |
|
|
lazy user |
send and receive parcel details to and from other companies |
minimize the amount of data inputs |
|
forgetful user |
be reminded of a parcel’s delivery date (if valid) |
in case I forget the date |
|
forgetful user |
view daily deliveries |
keep track of daily deliveries |
|
busy user |
add and remove tasks |
use addressbook as a task manager |
|
user |
store the sender and receiver addresses |
use these addresses as destinations/sources of my deliveries |
|
user |
share details with contacts with a specific tag |
minimize chance of someone else seeing them by accident |
|
user |
assign contacts and locations to tasks |
link my tasks with people and places |
|
user |
assign an expiry date to tasks |
tasks are deleted automatically |
|
user |
filter parcels by tags |
view specific parcels that are assigned with specific tags |
|
user |
filter tasks according to location |
be notified of deliveries I have at a specific location |
|
user |
retrieve my exact location on my device |
remember the current address and store my location |
|
user with a changing schedule |
edit created tasks |
change the details of task |
|
delivery man |
be able to check the status of my deliveries on the go |
|
|
delivery man |
be able to update the status of my deliveries on the go |
|
|
delivery man |
be able to check the address of the delivery I have to make on the go |
|
|
delivery man |
generate deliver route based on my list of deliveries |
know schedule for the day |
|
delivery man who travels a lot |
to know the shortest distance from one contact’s address to another |
|
|
delivery man who travels a lot |
set a reference location |
find the shortest distance from my reference location to a parcel’s delivery location |
|
new user |
input instructions into a chatbot interface |
I do not need to remember the format of commands |
|
user with many parcels in the address book |
sort parcels by name |
locate a parcel easily |
Appendix B: Use Cases
(For all use cases below, the System is the AddressBook
and the Actor is the user
, unless specified otherwise)
Use case: Delete parcel
MSS
-
User requests to list parcels
-
Ark shows a list of parcels and maximizes the
ParcelListPanel
in theMainWindow
UI -
User requests to delete a specific parcel in the list
-
Ark deletes the parcel
Use case ends
Extensions
-
2a. The list is empty
Use case ends
-
3a. The given index is invalid
-
3a1. Ark shows an error message.
Use case resumes at step 2
-
Use case: Add parcel
MSS
-
Use enters add command with the fields: name, tracking number, email, phone number, delivery date, delivery status and tags.
-
Ark validates that fields are correct.
-
Ark adds parcel
Use case ends
Extensions
-
2a. Ark detects errors in some fields.
-
2a1. Ark shows an error message.
Use case ends
-
-
1a. The user does not input an email.
-
1a1. Ark adds parcel with
NIL
in the email field.Use case ends
-
-
1b. The user does not input a phone number.
-
1b1. Ark adds parcel with
NIL
in the phone number field.Use case ends
-
-
1c. The user does not input a status.
-
1c1. Ark adds parcel with
PENDING
in the status field.Use case ends
-
Use case: Add parcel by prompt
MSS
-
User requests to add parcels without further details
-
Ark prompts user to input parcel identification number of parcel to add
-
User inputs identification number as requested
-
Ark prompts user to input name of recipient of parcel to add
-
User inputs name of recipient as requested
-
Ark prompts user to input phone number of recipient of parcel to add
-
User inputs phone number as requested
-
Ark prompts user to input email of recipient of parcel to add
-
User inputs email as requested
-
Ark prompts user to input delivery address of parcel to add
-
User inputs address as requested
-
Ark prompts user to input tags of parcel to add
-
User inputs tags as requested [optional]
-
Ark adds parcel
Use case ends
Extensions
-
3a. The user does not input a parcel identification number
-
3a1. Ark shows an error message
Use case resumes at step 2
-
-
5a. The user does not input a name
-
5a1. Ark shows an error message
Use case resumes at step 4
-
-
7a. The user does not input a valid phone number
-
7a1. Ark shows an error message
Use case resumes at step 6
-
-
9a. The user does not input a valid email
-
9a1. Ark shows an error message
Use case resumes at step 8
-
-
11a. The user does not input a valid address
-
11a1. Ark shows an error message
Use case resumes at step 10
-
-
13a. The user does not input a tag
-
13a1. Ark shows that no tag has been entered
Use case resumes at step 14
-
-
14. Ark shows error message if same parcel found
Use case ends
Use case: Understanding Delivery Dates
MSS
-
User adds a parcel with valid inputs and with
today
as input after delivery date prefixd/
-
Ark recognises the intent
-
Ark adds parcel with the current date on the user’s machine
Use case ends
Extensions
-
1a. The user inputs an more complicated date query such as
the week before christmas eve
-
1a1. Ark recognises the intent
-
1a2. Ark adds parcel with the date 17-12-2017, with the year being the current year
Use case ends
-
-
1b. The user inputs an invalid date query such as
pen pineapple apple pen
-
1b1. Ark does not recognise the intent
-
1b2. Ark shows an error message
Use case ends
-
Use case: Upload image of Parcel from local files
MSS
-
User requests to list parcels
-
Ark shows a list of parcels and maximizes the
ParcelListPanel
in theMainWindow
UI -
User requests to upload image of a specific parcel in the list
-
Ark prompts for location of image
-
User inputs file path
-
Ark updates image
Use case ends
Extensions
-
2a. The list is empty
Use case ends.
-
6a. The file path given is invalid
-
6a1. Ark shows an error message
Use case resumes at step 4
-
-
6b. The file type of file given is invalid
-
6b1. Ark shows an error message
Use case resumes at step 4
-
Use case: Set user reference location
MSS
-
User requests to set reference location
-
Ark updates reference location
Use case ends
{More to be added}
Appendix C: Non Functional Requirements
-
Should work on any mainstream OS as long as it has Java
1.8.0_60
or higher installed. -
Should be able to hold up to 1000 parcels without a noticeable sluggishness in performance for typical usage.
-
A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
-
Each Command should take at most 1 second to finish execution.
-
Should be able to handle any valid or invalid user input.
-
Should back up data inside the address book each time the user makes changes to the data.
-
Commands that do not require internet connection should still work when the user is not connected to the internet.
-
Should come with automated unit tests.
-
A new user should be able to use basic commands like add and delete without needing to refer to the help window after their first time using the application.
-
Should allow the user to upload images of any mainstream image format.
-
Hash String of the users personal contact information should only be made up of alphanumeric characters.
-
Should update the map automatically when the user changes their starting location.
{More to be added}
Appendix D: Glossary
Mainstream OS
Windows, Linux, Unix, OS-X
Appendix E: Product Survey
Table 1. Swift, reviewed by A. Pen Gwyn
Pros |
Cons |
Clean UI |
Cannot keep track of how parcel is handled e.g Fragile |
Automated dispatching |
Expensive |
Proprietary batching algorithm |
Table 2. Parcel Management Software, reviewed by P. Tato
Pros |
Cons |
Able to keep track of large amounts of data |
Poor UI |
Fast and Reliable |
Cannot add multiple parcels quickly |
Able to generate delivery routes |
Table 3. Journey, reviewed by John Prodman
Pros |
Cons |
Clean and Intuitive UI |
Slow response time |
Automated delivery scheduling |